How to use both Tailwind and Styled Components in Next JS
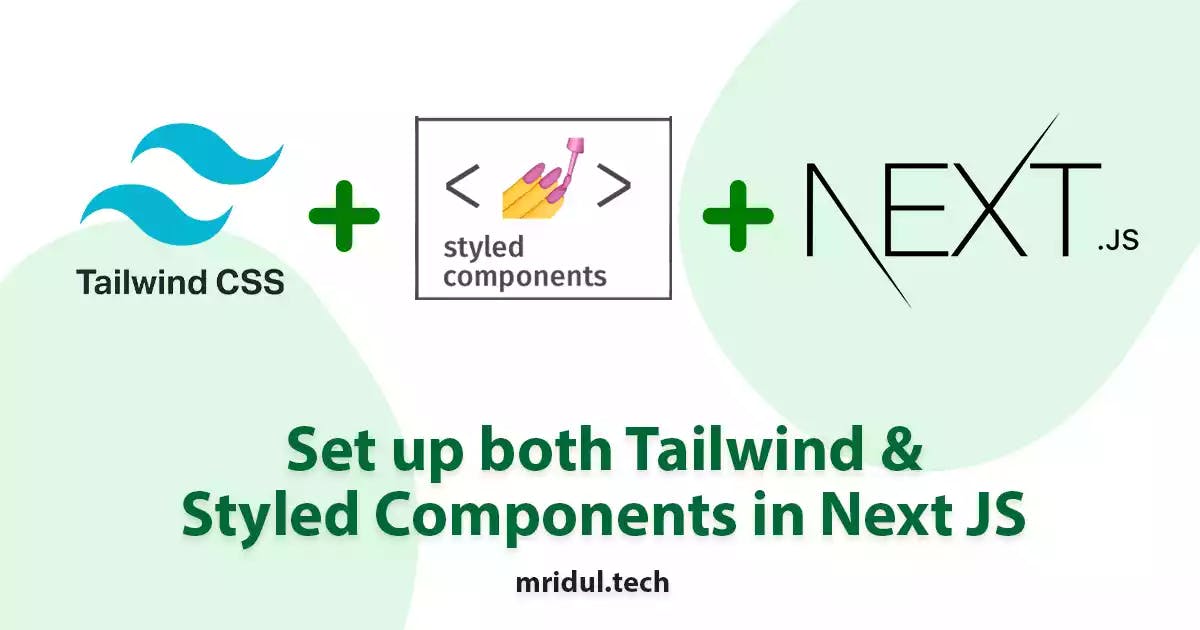
Styling is important in every website but it is equally difficult to add CSS with just .css
files. And when you’re using Next JS then you have so many options to choose from. But they are not easy to set up. Next JS
supports CSS modules by default but CSS modules are not that interesting to use. Even if we can not use nested selectors for styles. And we also need to add className
for the different components. This is a real headache for the developers to set different classes and maintain them with styles. But the interesting thing is we can set styles in so many ways. We can Tailwind CSS for styling. Tailwind has so many utility-based classes, which means we don’t need to maintain custom CSS classes for styling. And these utility-based classes are so much customizable that we will never get a similar layout as we get in the case of bootstrap. We have another way to add styles and that is styled-components. We can make normal HTML tags as styled-components and even make custom package tags as styled-componnets. styled-components adds styles for older browsers in the compilation time. So our website can look the same in all browsers. So let’s set up both Tailwind and Styled Components in Next JS
Creating a New Next JS Project
We will be creating a new Next JS project to set it up from scratch. For this article, we will give it a name tailwind-styled
For this, we will run the following command.
npx create-next-app tailwind-styled
#or
yarn create-next-app tailwind-styled
cd tailwind-styled
Tailwind CSS set up in Next JS
1. First, we will install the Tailwind CSS packages. We will install tailwindcss
and its peer dependencies
npm install -D tailwindcss postcss autoprefixer
2. In the next step, we have to run the init command to generate both tailwind.config.js
and postcss.config.js
.
npx tailwindcss init -p
3. You will see tailwind.config.js
at the root of your project. Open that file and add the following commands.
module.exports = {
content: [
"./pages/**/*.{js,ts,jsx,tsx}",
"./components/**/*.{js,ts,jsx,tsx}",
// Or if using `src` directory:
"./src/**/*.{js,ts,jsx,tsx}",
],
theme: {
extend: {},
},
plugins: [],
}
Also Read: How to add Google AdSense in Next JS
4. Make a global.css
file ./styles/global.css
and add the @tailwind
directives for each of Tailwind’s layers to it.
// ./styles/global.css
@tailwind base;
@tailwind components;
@tailwind utilities;
5. Import this global.css
in your _app.js
file. So that the tailwind classes are available for every file.
import '../styles/tailwind.css';
Now to check if the Tailwind is set up in done currently we can classNames
into a component
import React from 'react';
const Home = () => {
return (
<h1 className="text-3xl font-bold underline">
Hello world!
</h1>
);
};
export default Home;
If you want to use SCSS with Tailwind in Next JS you can check out How to use SCSS with Tailwind in Next JS
Styled Components set up in Next JS
1. For `styled-components`, we need to install the following package with the command below
npm i styled-compoments
or
yarn add styled-compoments
2. Next JS uses Server Side Rendering so if you add styles with styled-components it will load lazily. And our website will look wired at first. For this, we need to add styled-components on the server side. We can use two methods. let’s check them one by one
With Babel: We have to add a few babel configurations we can add server-side styles. for that, we have to install babel-plugin-styled-components
as a dev dependency.
npm install --save-dev babel-plugin-styled-components
After that add a .babelrc
file in your root directory. And add the following configurations.
{
"presets": ["next/babel"],
"plugins": [["styled-components", { "ssr": true }]]
}
With Next Config: With Next JS v13.1.0 we compile the styled-components directly with next.config.js
. We just need to add some configuration in our next config file.
// next.config.js
module.exports = {
compiler: {
styledComponents: true
}
}
Also Read: Next JS Project Ideas to Boost Your Portfolio
3. This step is mandatory for both methods. We need to add these functions to compile styles on the server side. Open _document.js
file or create one if you don’t have one.
import Document from 'next/document'
import { ServerStyleSheet } from 'styled-components'
export default class MyDocument extends Document {
static async getInitialProps(ctx) {
const sheet = new ServerStyleSheet()
const originalRenderPage = ctx.renderPage
try {
ctx.renderPage = () =>
originalRenderPage({
enhanceApp: (App) => (props) =>
sheet.collectStyles(<App {...props} />),
})
const initialProps = await Document.getInitialProps(ctx)
return {
...initialProps,
styles: (
<>
{initialProps.styles}
{sheet.getStyleElement()}
</>
),
}
} finally {
sheet.seal()
}
}
}
Now let’s test with one styled-components in our Next JS app.
import React from 'react';
import styled from 'styled-components';
const Home = () => {
return <Heading>Hello world!</Heading>;
};
export default Home;
const Heading = styled.h1`
font-size: 2rem;
font-weight: 800;
text-decoration: underline;
`;
Use both Tailwind and Styled Components in Next JS Component
We can also add Tailwind classes into a styled-component. if we add the same style with Tailwind and styled-component then the styled-component styles will get the priority. Let’s look at a component where we can apply both Tailwind and Styled Components
import React from 'react';
import styled from 'styled-components';
const Home = () => {
return (
<StyledHeading className="text-green-800 underline">
Hello world!
</StyledHeading>
);
};
export default Home;
const StyledHeading = styled.h1`
font-size: 2rem;
font-weight: 800;
`;
That’s it. This is how you can set up and use both Tailwind and Styled Components in the same Next JS Project. If you’re looking for an example project, here is a Next JS starter template with Tailwind and Styled Components. You can even use it as a template with the following
// WIth NPM
npx create-next-app . -e https://github.com/Mridul2820/next-template
// With YARN
yarn create-next-app . -e https://github.com/Mridul2820/next-template
You may also like
How to add Google Web Stories in Next JS

Dec 14, 2023
·10 Min Read
In the fast-paced digital world, user engagement is key to the success of any website. One effective way to captivate your audience is by incorporating Google Web Stories into your Next JS website. These visually appealing and interactive stories can make your content more engaging and shareable. In this comprehensive guide, we’ll walk you through […]
Read More
How to send Emails in Next JS for Free using Resend
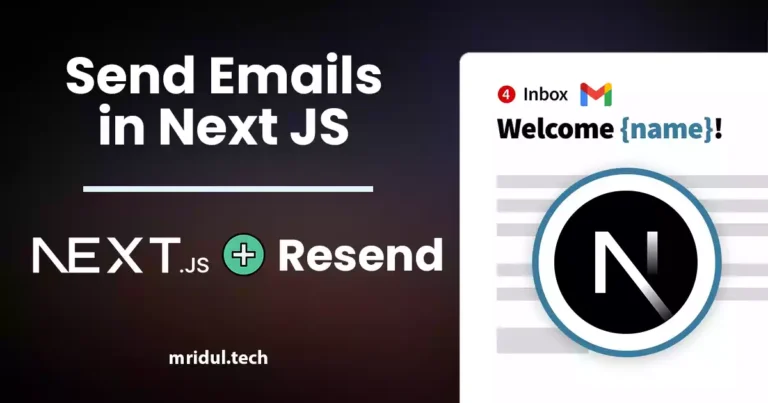
Nov 10, 2023
·7 Min Read
Sending emails in web applications is a crucial feature, and in this article, we will explore how to send Emails in Next JS for free using Resend. Next JS is a popular framework for building React applications, and Resend is a handy tool for email integration. By the end of this guide, you’ll have the […]
Read More
How to add Google Login in Next.js with Appwrite

Nov 01, 2023
·7 Min Read
Are you looking to enhance user authentication in your Next.js application? Integrating Social Login with Appwrite can be a game-changer. Add Google Login to your Next.js app with Appwrite. This article will guide you through the process, and practical tips to add Google Login in Next.js with Appwrite. GitHub Code: Google Login in Next.js with […]
Read More
JavaScript Project Ideas to Boost Your Portfolio

Oct 13, 2023
·3 Min Read
JavaScript is the backbone of web development, and mastering it is essential for any aspiring developer. While learning the basics is crucial, building real-world projects is the key to solidifying your knowledge. In this comprehensive guide, we’ll present a diverse range of JavaScript project ideas that cater to different skill levels and interests. These projects […]
Read More
How to Generate robots.txt in Next JS?

Oct 05, 2023
·4 Min Read
In the world of web development and search engine optimization (SEO), ensuring that your website is easily accessible and properly indexed by search engines is paramount. One essential tool that aids in this process is the creation of a robots.txt file. In this comprehensive guide, we, the experts, will walk you through the process of […]
Read More
How to Generate Sitemap in Next JS?
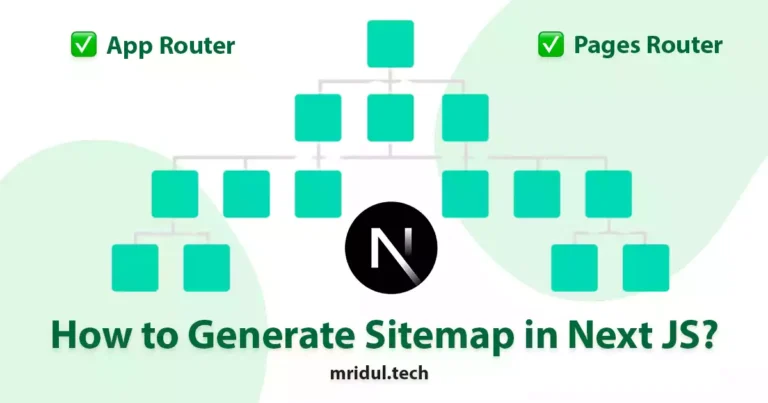
Sep 28, 2023
·8 Min Read
In today’s digital landscape, optimizing your website’s SEO is crucial to attracting more organic traffic and ranking higher on search engine result pages (SERPs). One essential aspect of SEO is creating a sitemap, which helps search engines crawl and index your website efficiently. If you’re using Next JS for your website development, this guide will […]
Read More