How to Generate Sitemap in Next JS?
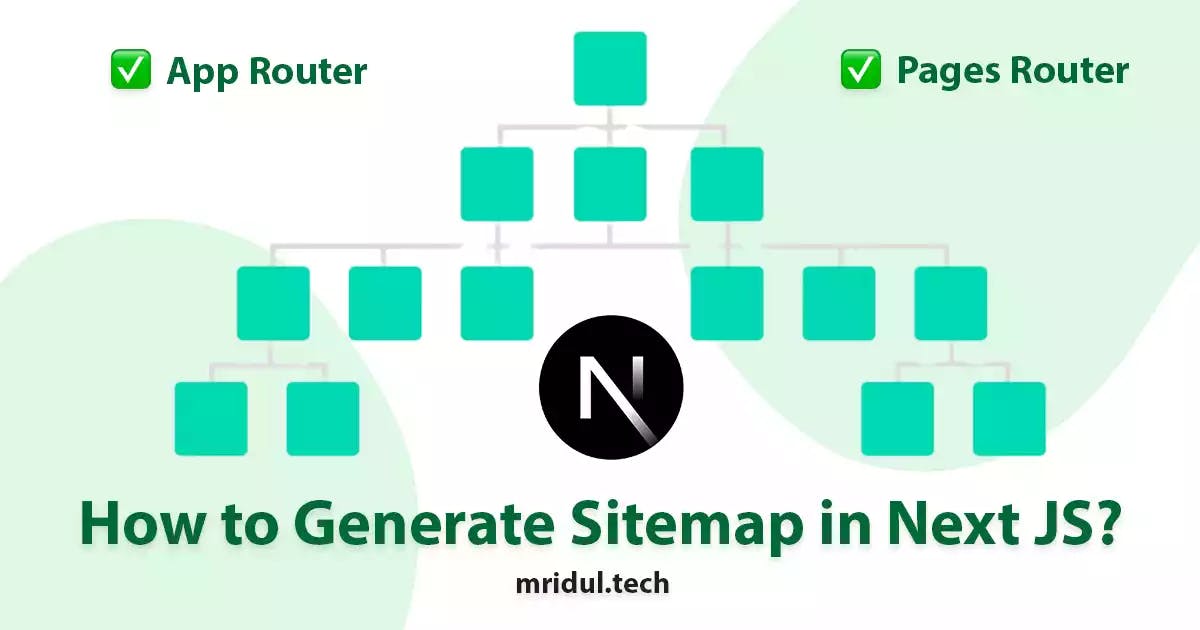
In today’s digital landscape, optimizing your website’s SEO is crucial to attracting more organic traffic and ranking higher on search engine result pages (SERPs). One essential aspect of SEO is creating a sitemap, which helps search engines crawl and index your website efficiently. If you’re using Next JS for your website development, this guide will walk you through generating a sitemap in Next JS. Let’s dive into the world of sitemaps and SEO.
Understanding the Importance of Sitemaps
A sitemap is essentially a roadmap for search engines, guiding them through the intricate web of your website. Here’s why it matters:
- Enhances Search Engine Crawling: A well-structured sitemap ensures that search engine bots can easily discover and index your web pages. This means more of your content gets included in search engine results.
- Improved User Experience: Sitemaps are not just for search engines; they also benefit your visitors. Users can navigate your site more efficiently, finding the content they need with ease
- Boosts SEO Ranking: When search engines can easily crawl and index your pages, it positively impacts your SEO ranking. A sitemap is a must for climbing the search engine results ladder.
Generate Sitemap in Next JS in Pages Router
Let’s start by talking about how to add a sitemap to a website that uses the pages
directory.
Step 1 – Create the sitemap Function
In the pages folder, first, create a sitemap.xml.js
file, then add a default SiteMap component to it.
src/
pages/
sitemap.xml.js
// pages/sitemap.xml.js
export default function SiteMap() {}
When the sitemap.xml
endpoint is accessed, this component’s primary function is to render the sitemap page. The getServerSideProps
function, which will obtain the URLs for each post, call the sitemap rendering function, and publish the response with the text/xml
content-type header, will provide the real content.
Also Read: How to create a URL Shortener with Next JS and Sanity
Step 2 – Generate the Sitemap with Static Links
We return an empty props object for the SiteMap component since the goal of getServerSideProps
in this case is to deliver a customised response along with the sitemap when the /sitemap.xml URL is requested. Our API for obtaining sorted blog articles is called getSortedPostsData.
// pages/sitemap.xml.js
const homepage = "https://www.mridul.tech"
function generateSiteMap() {
return `<?xml version="1.0" encoding="UTF-8"?>
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9">
<url>
<loc>${homepage}</loc>
<lastmod>${new Date().toISOString()}</lastmod>
</url>
<url>
<loc>${homepage}/abous-us</loc>
<lastmod>${new Date().toISOString()}</lastmod>
</url>
<url>
<loc>${homepage}/contact-us</loc>
<lastmod>${new Date().toISOString()}</lastmod>
</url>
</urlset>
`;
}
export async function getServerSideProps({ res }) {
const sitemap = generateSiteMap();
res.setHeader("Content-Type", "text/xml");
res.write(sitemap);
res.end();
return {
props: {},
};
}
export default function SiteMap() {}
Here we have added 3 static links. The homepage, about-us page and contact-us page.
Also Read: Next JS Project Ideas to Boost Your Portfolio
Step 3 – Adding Dynamic links in the Sitemap
First, we will fetch all the posts we want to add to our sitemap. Fatch your blogs from your backend API and pass it to the generateSiteMap
function. Now map the post array and return the full blog URL. And finally, after the static URLs in the sitemap map the dynamic sitemap. That’s it!
const homepage = "https://www.mridul.tech";
function generateSiteMap(data) {
const urls = data?.map((item) => {
const slug = item?.slug;
return {
url: `${homepage}/blogs/${slug}`,
};
});
return `<?xml version="1.0" encoding="UTF-8"?>
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9">
<url>
<loc>${homepage}</loc>
<lastmod>${new Date().toISOString()}</lastmod>
</url>
<url>
<loc>${homepage}/abous-us</loc>
<lastmod>${new Date().toISOString()}</lastmod>
</url>
<url>
<loc>${homepage}/contact-us</loc>
<lastmod>${new Date().toISOString()}</lastmod>
</url>
${urls
?.map((item: any) => {
return (
item &&
`<url>
<loc>${item?.url}</loc>
<lastmod>${new Date().toISOString()}</lastmod>
</url>
`
);
})
.join("")}
</urlset>
`;
}
export async function getServerSideProps({ res }) {
const blogs = await fetch("https://www.someapi.com/posts");
const data = await blogs.json();
const sitemap = generateSiteMap(data);
res.setHeader("Content-Type", "text/xml");
res.write(sitemap);
res.end();
return {
props: {},
};
}
export default function SiteMap() {}
Generate Sitemap in Next JS in App Router(13.3 and higher)
The file-based metadata API was added to Next.js version 13.3. And makes it easier to work with page metadata by exporting a Metadata object. In addition to making static and dynamic sitemap management simpler, this also does away with the necessity for manual sitemap generation.
This gives us the opportunity to develop a sitemap.js file that takes care of the majority of the repetitive logic needed to create the sitemap for the website.
app/
sitemap.js
Our primary goal in the sitemap.js file is to map the posts and static URLs to the properties of an object called a Sitemap, and then to return that object.
// app/sitemap.js
const homepage = "https://www.mridul.tech";
export default async function sitemap() {
const blogs = await fetch("https://www.someapi.com/posts");
const data = await blogs.json();
const posts = data.map((post) => ({
url: `${homepage}/blogs/${post.slug}`,
lastModified: new Date(post.date).toISOString(),
}));
const routes = ["", "/about-us", "/contact-us"].map((route) => ({
url: `${homepage}${route}`,
lastModified: new Date().toISOString(),
}));
return [...routes, ...posts];
}
To display the date of the most recent alteration for each page, we also include the lastModified
field. When scanning and indexing your website, search engines may find this information useful.
Generate Sitemap in Next JS in App Router(13.2 and lower)
In Next.js 13.2 and earlier, we can achieve the same behaviour by using Route Handlers. Using the Web Request and Response APIs, route handlers enable the development of unique request handlers for particular routes. They take the place of the pages
directory’s API Routes.
Our sitemap contains non-UI material, which is something that route handlers may also utilise to return, according to the documentation, which we have read in greater detail. In order to define Route Handlers, the sitemap.xml.js file must first be moved into the app directory and given the new name route.js. We’ll nest it inside the sitemap.xml folder as well.
The app
directory structures is contrasted below:
app/
sitemap.xml/
route.js
The route.js file will then be modified to add a special GET request handler for this route, which will return a special response along with the created sitemap. We’ll also get rid of the Sitemap component and the getServerSideProps
function as we no longer require them.
const homepage = "https://www.mridul.tech";
function generateSiteMap(data) {
const urls = data?.map((item) => {
const slug = item?.slug;
return {
url: `${homepage}/blogs/${slug}`,
};
});
return `<?xml version="1.0" encoding="UTF-8"?>
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9">
<url>
<loc>${homepage}</loc>
<lastmod>${new Date().toISOString()}</lastmod>
</url>
<url>
<loc>${homepage}/abous-us</loc>
<lastmod>${new Date().toISOString()}</lastmod>
</url>
<url>
<loc>${homepage}/contact-us</loc>
<lastmod>${new Date().toISOString()}</lastmod>
</url>
${urls
?.map((item: any) => {
return (
item &&
`<url>
<loc>${item?.url}</loc>
<lastmod>${new Date().toISOString()}</lastmod>
</url>
`
);
})
.join("")}
</urlset>
`;
}
export async function GET() {
const blogs = await fetch("https://www.someapi.com/posts");
const data = await blogs.json();
const sitemap = generateSiteMap(data);
return new Response(sitemap, {
status: 200,
headers: {
"Cache-control": "public, s-maxage=86400, stale-while-revalidate",
"content-type": "application/xml",
},
});
}
Here, we also include the stale-while-revalidate and s-maxage of 1-day directives. You can get more information about them on MDN.
After making these modifications, go to https://yoursite.com/sitemap.xml. Now you will see the most recent sitemap, which is produced from the app
directory.
Also Read: How to Build PWA with Next JS?
Validate Your Sitemap created in Next JS
Once you’ve generated your sitemap, it’s essential to validate it to ensure it meets the required standards. Follow these steps:
Step 1: Use Online Sitemap Validators: There are several online tools and validators available. These can check the validity of your sitemap. Simply upload your sitemap or provide its URL to get a detailed report.
Example: To validate the sitemap you created in Next JS, visit xml-sitemaps.com.
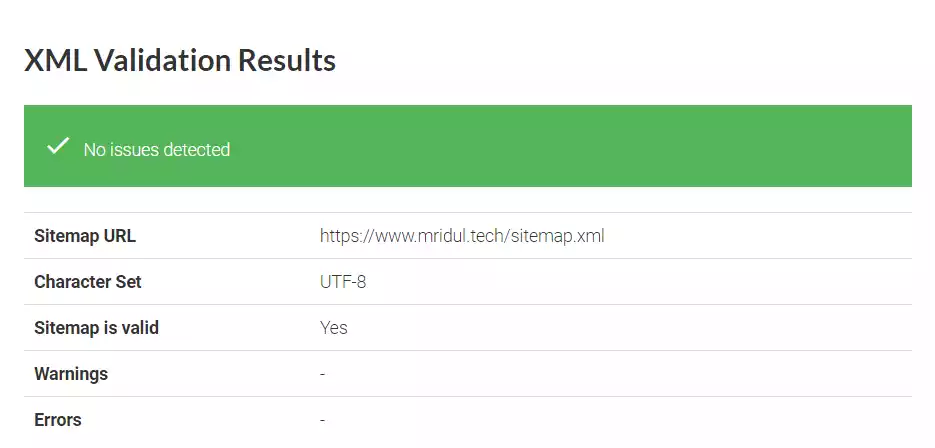
Step 2: Address Errors: If the validator detects any errors or issues in your sitemap, take the time to address them. Common issues include broken links and incorrect URL structures.
Step 3: Revalidate: After making the necessary corrections, revalidate your sitemap to ensure it now complies with the standards.
Conclusion: Generate Sitemap in Next JS
Generating a sitemap in Next JS is a crucial step in optimizing your website for search engines and improving user experience. By following the steps outlined in this guide, including validating your sitemap, you’re well on your way to boosting your SEO efforts. Remember, a well-structured sitemap is like a roadmap. It leads both search engines and users to the treasure trove of your website’s content.
You may also like
How to add Google Web Stories in Next JS

Dec 14, 2023
·10 Min Read
In the fast-paced digital world, user engagement is key to the success of any website. One effective way to captivate your audience is by incorporating Google Web Stories into your Next JS website. These visually appealing and interactive stories can make your content more engaging and shareable. In this comprehensive guide, we’ll walk you through […]
Read More
How to send Emails in Next JS for Free using Resend
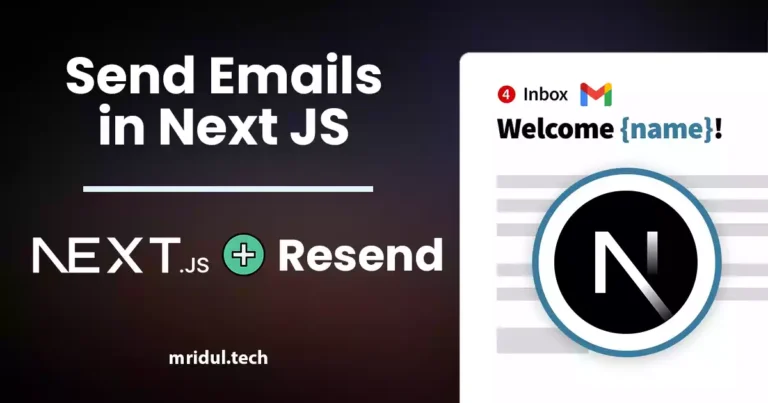
Nov 10, 2023
·7 Min Read
Sending emails in web applications is a crucial feature, and in this article, we will explore how to send Emails in Next JS for free using Resend. Next JS is a popular framework for building React applications, and Resend is a handy tool for email integration. By the end of this guide, you’ll have the […]
Read More
How to add Google Login in Next.js with Appwrite

Nov 01, 2023
·7 Min Read
Are you looking to enhance user authentication in your Next.js application? Integrating Social Login with Appwrite can be a game-changer. Add Google Login to your Next.js app with Appwrite. This article will guide you through the process, and practical tips to add Google Login in Next.js with Appwrite. GitHub Code: Google Login in Next.js with […]
Read More
JavaScript Project Ideas to Boost Your Portfolio

Oct 13, 2023
·3 Min Read
JavaScript is the backbone of web development, and mastering it is essential for any aspiring developer. While learning the basics is crucial, building real-world projects is the key to solidifying your knowledge. In this comprehensive guide, we’ll present a diverse range of JavaScript project ideas that cater to different skill levels and interests. These projects […]
Read More
How to Generate robots.txt in Next JS?

Oct 05, 2023
·4 Min Read
In the world of web development and search engine optimization (SEO), ensuring that your website is easily accessible and properly indexed by search engines is paramount. One essential tool that aids in this process is the creation of a robots.txt file. In this comprehensive guide, we, the experts, will walk you through the process of […]
Read More
Node JS Project Ideas to Boost Your Portfolio
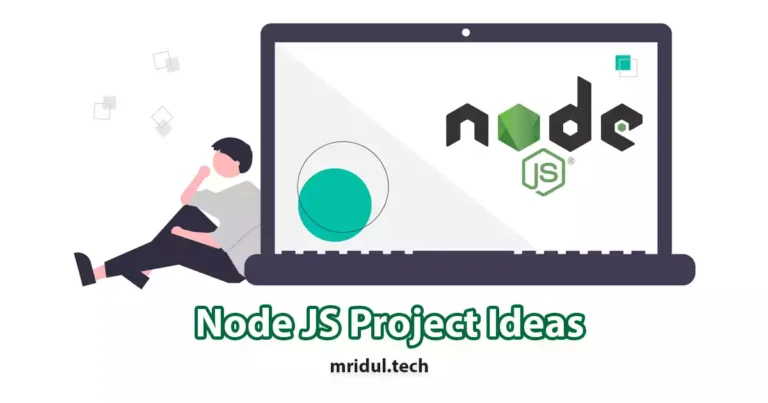
Sep 20, 2023
·4 Min Read
In the ever-evolving world of technology, staying competitive and relevant is key. As a developer, enhancing your skills and expanding your portfolio is essential. Node.js, with its versatility and wide range of applications, is an excellent choice to boost your portfolio. In this article, we will explore 10 Node JS project ideas that not only […]
Read More