How to handle Cookie Consent in Next JS

In order to comply with GDPR and other European regulations, the majority of websites nowadays must include a cookie consent system. This basically implies that you cannot place cookies on a user’s computer without that user’s consent. Additionally, you cannot let other services (such as analytics or advertising) that are active on your website store cookies on your domain. This is a guide on How to Handle Cookie Consent in Next JS easily.
Preview of Cookie Consent
By default, the Cookie Consent looks like this. But we can change the background colour, and text colour and add extra buttons. In fact, we can add handlers for accept and decline buttons.

Install-Package for Cookie Consent in Next JS
To easily manage Cookie Consent we will be using a package that will handle the process under the hood and the package is highly customizable. The package is react-cookie-consent by Mastermindzh. It has 66k+ downloads in NPM so we can trust this package.
npm install react-cookie-consent
or use yarn:
yarn add react-cookie-consent
Also Read: How to Generate ZIP with File Links in Next JS
How to add the Cookie Consent
First, we need to import react-cookie-consent
package like this
import CookieConsent from "react-cookie-consent";
You can use this React component in your _app.js
then it will be available for all pages Basic Usage:
<CookieConsent>This website uses cookies to enhance the user experience.</CookieConsent>
We can use the package with many available props and customize the Cookie Consent banner. We can even change the button text on the button. Here is an example –
import React from 'react';
import CookieConsent from 'react-cookie-consent';
const CookieAccept = () => {
return (
<CookieConsent
enableDeclineButton
flipButtons
location="bottom"
buttonText="I understand"
cookieName="YourCoockieName"
style={{ background: 'blue' }}
buttonStyle={{
color: '#000',
fontSize: '15px',
}}
declineButtonStyle={{
margin: '10px 10px 10px 0',
}}
expires={450}
>
This website uses cookies
</CookieConsent>
);
};
export default CookieAccept;
With this, you can get a consent banner like this –

Also Read: Save Form data in Google Sheets with Next JS
Customize the Cookie Consent
Available props for cookie consent
There are so many props available to customize the consent banner.
- location: We can change the position of the banner with
location
a prop. It will accept a string, “top”, “bottom” or “none”. The default value is “bottom” - cookieName: This is the Name of the cookie used to track whether the user has agreed. You should use a different name for every website.
- enableDeclineButton: Decline Button is disabled by default. But we can use this prop to enable this.
- flipButtons: This prop can be used for flipping the accept and decline button.
- buttonText: Accept button text
- declineButtonText: Text to appear on the decline button
You can check all the props available in the GitHub Repository
Also Read: How to Generate ZIP with File Links in Next JS and React JS
Accept and Decline handle functions
After the user clicks the accept button, a function named onAccept
is one of the properties that will be invoked. It is called with an object that has the boolean attribute acceptedByScrolling
to specify if the user’s scrolling caused the acceptance. You can offer the following function:
<CookieConsent
onAccept={(acceptedByScrolling) => {
if (acceptedByScrolling) {
// triggered if user scrolls past threshold
alert("Accept was triggered by user scrolling");
} else {
alert("Accept was triggered by clicking the Accept button");
}
}}
/>
The onDecline
prop function, which is called after the user clicks the decline button, may be utilised if the decline button is enabled. You may turn on the button and give it the following functionality:
<CookieConsent
enableDeclineButton
onDecline={() => {
alert("nay!");
}}
/>
Also Read: How to use both Tailwind and Styled Components in Next JS
Debugging Cookie Consent
When we accept the cookie content the content disappears that why it’s hard to debug. But we can add debug={true}
prop in order to enable the banner again.
<CookieConsent debug={true}></CookieConsent>
Also Read: How to Add Google Analytics in NextJS
Getting the cookie value in the code
getCookieConsentValue is a function that react-cookie-consent exports. It can be used in your own code as follows:
import CookieConsent, {
Cookies,
getCookieConsentValue,
} from 'react-cookie-consent';
console.log(getCookieConsentValue());
Reset the Cookie Consent
We can use resetCookieConsentValue
to reset the react-cookie-consent
and remove the older cookie from the client site.
import CookieConsent, {
Cookies,
resetCookieConsentValue,
} from 'react-cookie-consent';
console.log(resetCookieConsentValue());
You may also like
How to add Styled components in Next.js App router

May 11, 2024
·4 Min Read
Styled components have become a popular choice for styling React applications due to their simplicity and flexibility. When it comes to integrating styled components into a Next.js application, it’s essential to understand how to leverage them effectively within the app router. In this guide, we’ll explore step-by-step how to add styled components to a Next.js […]
Read More
How to add Google Web Stories in Next JS

Dec 14, 2023
·10 Min Read
In the fast-paced digital world, user engagement is key to the success of any website. One effective way to captivate your audience is by incorporating Google Web Stories into your Next JS website. These visually appealing and interactive stories can make your content more engaging and shareable. In this comprehensive guide, we’ll walk you through […]
Read More
How to send Emails in Next JS for Free using Resend
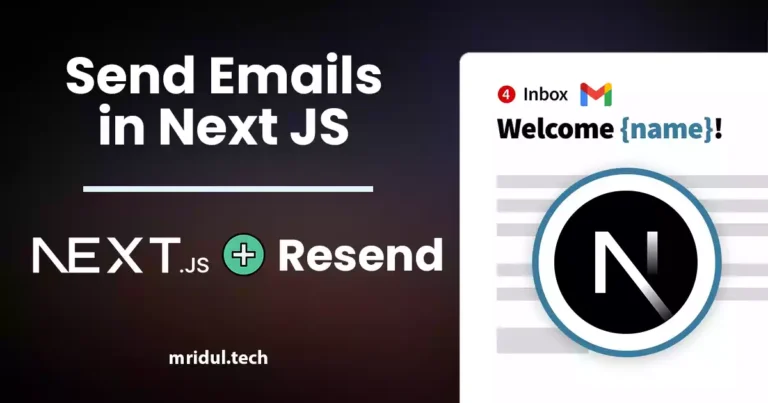
Nov 10, 2023
·7 Min Read
Sending emails in web applications is a crucial feature, and in this article, we will explore how to send Emails in Next JS for free using Resend. Next JS is a popular framework for building React applications, and Resend is a handy tool for email integration. By the end of this guide, you’ll have the […]
Read More
How to add Google Login in Next.js with Appwrite

Nov 01, 2023
·7 Min Read
Are you looking to enhance user authentication in your Next.js application? Integrating Social Login with Appwrite can be a game-changer. Add Google Login to your Next.js app with Appwrite. This article will guide you through the process, and practical tips to add Google Login in Next.js with Appwrite. GitHub Code: Google Login in Next.js with […]
Read More
How to add Protected Routes in Next JS

Oct 28, 2023
·5 Min Read
In the world of web development, security is paramount. Whether you are building a simple blog or a complex web application, protecting certain routes and pages from unauthorized access is a crucial step. In this comprehensive guide, we will walk you through the process of adding protected routes in Next JS, ensuring that your web […]
Read More
How to run localhost 3000 on https in Next JS

Oct 20, 2023
·2 Min Read
In the ever-evolving world of web development, having a secure local development environment is crucial. If you’re working with Next.js and need to run localhost on HTTPS with port 3000, you’re in the right place. In this comprehensive guide, we will walk you through the process step by step, ensuring you have a secure and […]
Read More