How to add Protected Routes in Next JS
Mridul Panda
Oct 28, 2023
·5 Min Read
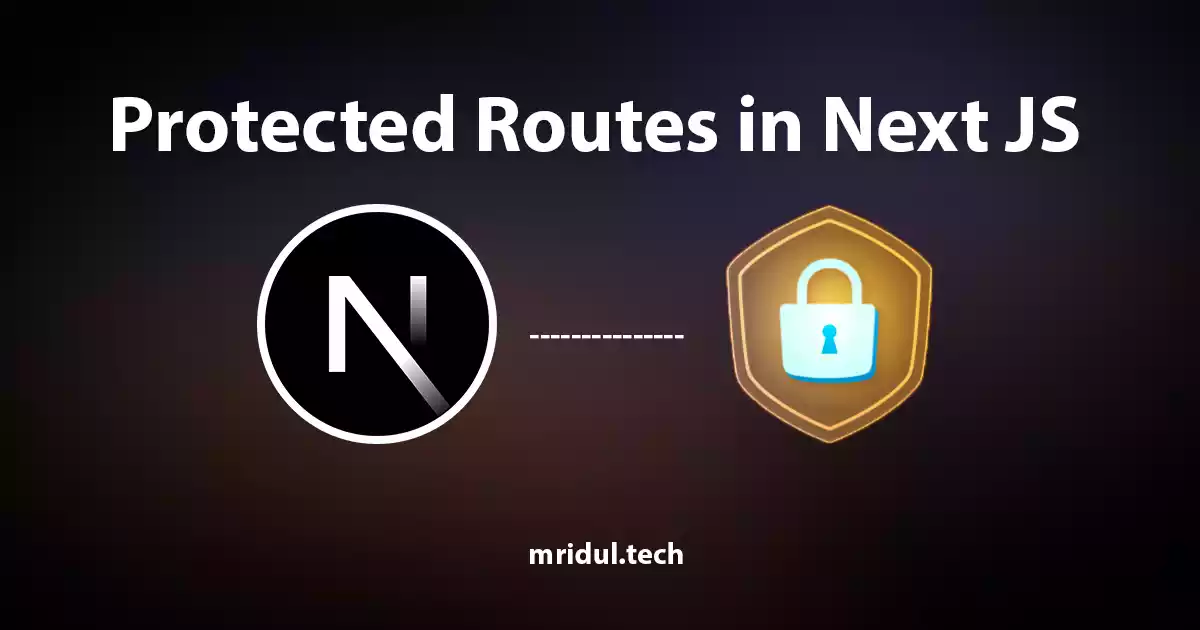
In the world of web development, security is paramount. Whether you are building a simple blog or a complex web application, protecting certain routes and pages from unauthorized access is a crucial step. In this comprehensive guide, we will walk you through the process of adding protected routes in Next JS, ensuring that your web application is secure and user-friendly.
What Are Protected Routes?
Before diving into the “how,” let’s clarify what protected routes are. Protected routes are web pages or sections of your application that require authentication or authorization to access. These routes are often restricted to logged-in users, ensuring that sensitive information remains confidential.
Why Protected Routes Matter?
Protected routes offer several advantages:
- Enhanced Security: By limiting access to authenticated users, you reduce the risk of data breaches and unauthorized access.
- Personalized User Experience: Protected routes allow you to tailor the user experience, showing different content or features based on user roles.
- Compliance: In some cases, regulations like GDPR require you to protect certain user data. Protected routes help you meet these legal requirements.
How to Add Protected Routes in Next JS
Now that we understand the importance of protected routes, let’s get into the nitty-gritty of how to implement them in Next JS. Follow these steps carefully:
Step 1: Getting Started with Next.js
Create a New Next.js App: Create a new Next.js project by running the following command:
npx create-next-app my-next-app
Navigate to Your Project: Move to the newly created project directory:
cd my-next-app
Also Read: How to Use SVG in Next JS?
Step 2: Set Up Authentication
For this project, we are going to use a simple email and password Authentication. So go ahead and set up your Authentication process. We are also using the JWT token for the authorisation. The main thing here is to save your JWT or any token to the cookies.
Tip: Don’t use localstorage
to save the token as it can be accessed on the client side only. However, you can access the cookie on both the client and server side.
Step 3: Save the token in the cookie
To save the cookie we will use a package named js-cookie
. To install the package run the following command:
npm i js-cookie
Now we will save the token in the cookie after the success of sign-in. Here is an example:
import Cookies from "js-cookie";
// Signin Function
const signin = async (email, password) => {
// Dunnmy API Signin
const data = await fetch("/api/signin", {
method: "POST",
body: JSON.stringify({ email, password }),
headers: { "Content-Type": "application/json" },
});
const res = await data.json();
if (res.ok) {
// Set token to cookie
Cookies.set("token", res?.data?.token);
}
};
Also Read: How to Generate Sitemap in Next JS?
Step 4 – Add middleware to Create Protected Routes in Next JS
Now we have saved the token in the cookie, we can set up the protected route. Create a file named middleware.js
in the root of your Next JS Project. The code in this file will run first before every request.
Learn More: Next JS Middleware official Docs
// middleware.js
import { NextResponse } from 'next/server';
export default function middleware(req) {
let loggedin = req.cookies.get('token');
const { pathname } = req.nextUrl;
if (loggedin && pathname === '/signin') {
return NextResponse.redirect(new URL('/', req.url));
}
if (!loggedin && pathname !== '/signin') {
return NextResponse.redirect(new URL('/signin', req.url));
}
}
export const config = {
matcher: '/((?!api|static|.*\\..*|_next).*)',
};
Explanation of Next JS Middleware Code
- Import Statement: firstly, It imports the
NextResponse
object from thenext/server
module. - Middleware Function: The
middleware
function takes areq
parameter, which represents the incoming request. - Cookie Check: It first attempts to retrieve a cookie named ‘token’ from the incoming request’s cookies using
req.cookies.get('token')
. This likely checks if a user is logged in by checking for the presence of a ‘token’ cookie. - Request Pathname: Once It extracts the
pathname
from the request’snextUrl
object. Thepathname
represents the path part of the URL. - Conditional Redirects:
- If a user is logged in (
loggedin
is truthy) and the request path is ‘/signin’, it performs a redirect usingNextResponse.redirect
to the root URL (‘/’) while preserving any query parameters from the original request. - If a user is not logged in (
loggedin
is falsy) and the request path is not ‘/signin’, it redirects to the ‘/signin’ page while preserving the original request’s URL and query parameters.
- If a user is logged in (
- Configuration Object: Finally, The
config
object specifies amatcher
property with a regular expression pattern. This pattern is used to match which requests should go through this middleware. In this case, it appears to match all routes except those that contain ‘api’, ‘static’, any file extensions (like ‘.js’ or ‘.css’), and the ‘_next’ folder.
As a result, this middleware examines the request path and the presence of a “token” cookie to determine whether a user is logged in. The user is redirected to the proper page (either the root or the ‘/signin’ page) if the user’s login status and the requested route do not match, while the original URL and query parameters are preserved. The Next.js application probably uses this middleware to restrict access to particular routes.
Also Read: Next JS Project Ideas to Boost Your Portfolio
Conclusion
In this comprehensive guide, we’ve explored how to add a protected route in Next JS, enhancing the security of your web application. By following these steps and best practices, you can ensure that only authorized users can access sensitive content. Protecting your routes is a vital aspect of creating a secure and reliable Next JS application.
You may also like
How to add Styled components in Next.js App router
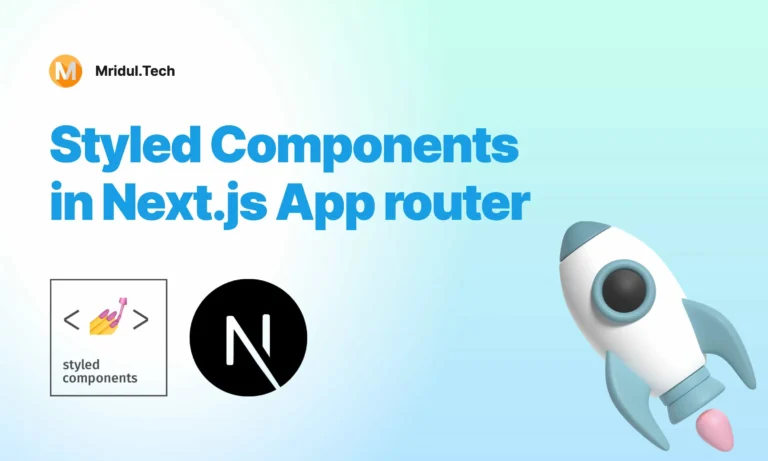
May 11, 2024
·4 Min Read
Styled components have become a popular choice for styling React applications due to their simplicity and flexibility. When it comes to integrating styled components into a Next.js application, it’s essential to understand how to leverage them effectively within the app router. In this guide, we’ll explore step-by-step how to add styled components to a Next.js […]
Read More
How to add Google Web Stories in Next JS
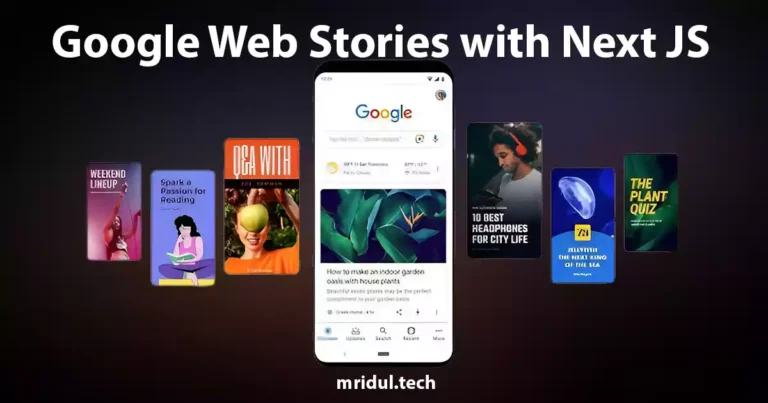
Dec 14, 2023
·10 Min Read
In the fast-paced digital world, user engagement is key to the success of any website. One effective way to captivate your audience is by incorporating Google Web Stories into your Next JS website. These visually appealing and interactive stories can make your content more engaging and shareable. In this comprehensive guide, we’ll walk you through […]
Read More
How to send Emails in Next JS for Free using Resend
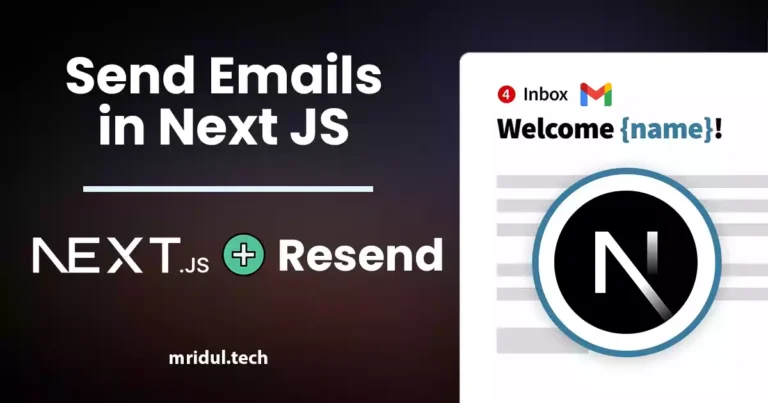
Nov 10, 2023
·7 Min Read
Sending emails in web applications is a crucial feature, and in this article, we will explore how to send Emails in Next JS for free using Resend. Next JS is a popular framework for building React applications, and Resend is a handy tool for email integration. By the end of this guide, you’ll have the […]
Read More
How to add Google Login in Next.js with Appwrite
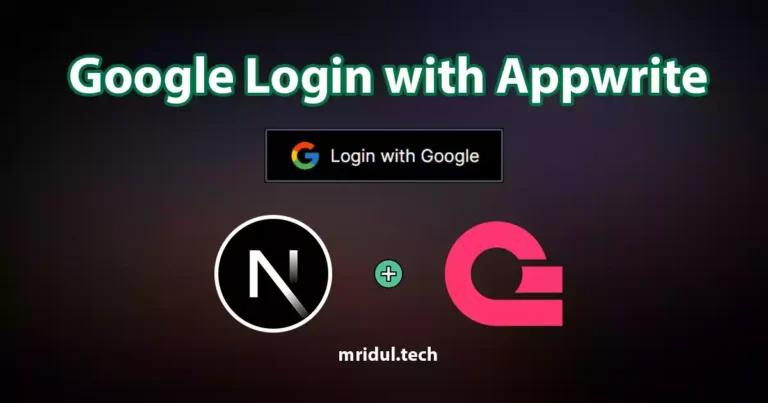
Nov 01, 2023
·7 Min Read
Are you looking to enhance user authentication in your Next.js application? Integrating Social Login with Appwrite can be a game-changer. Add Google Login to your Next.js app with Appwrite. This article will guide you through the process, and practical tips to add Google Login in Next.js with Appwrite. GitHub Code: Google Login in Next.js with […]
Read More
How to run localhost 3000 on https in Next JS
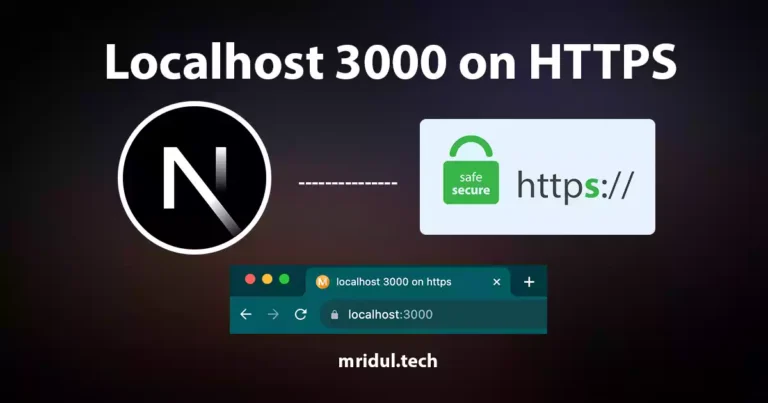
Oct 20, 2023
·2 Min Read
In the ever-evolving world of web development, having a secure local development environment is crucial. If you’re working with Next.js and need to run localhost on HTTPS with port 3000, you’re in the right place. In this comprehensive guide, we will walk you through the process step by step, ensuring you have a secure and […]
Read More
How to Generate robots.txt in Next JS?
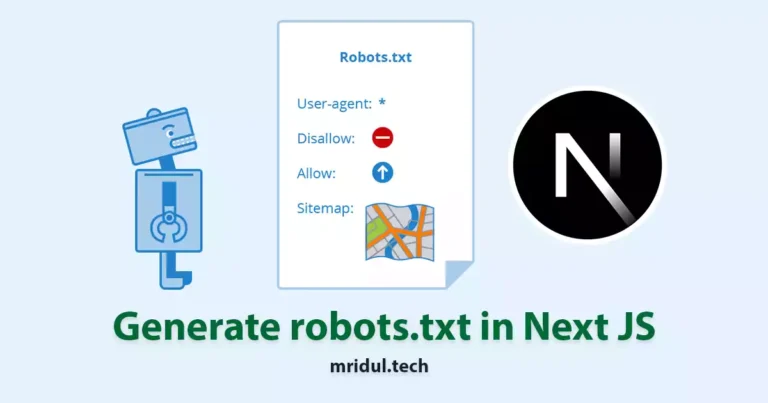
Oct 05, 2023
·4 Min Read
In the world of web development and search engine optimization (SEO), ensuring that your website is easily accessible and properly indexed by search engines is paramount. One essential tool that aids in this process is the creation of a robots.txt file. In this comprehensive guide, we, the experts, will walk you through the process of […]
Read More