How to create a URL Shortener with Next JS and Sanity
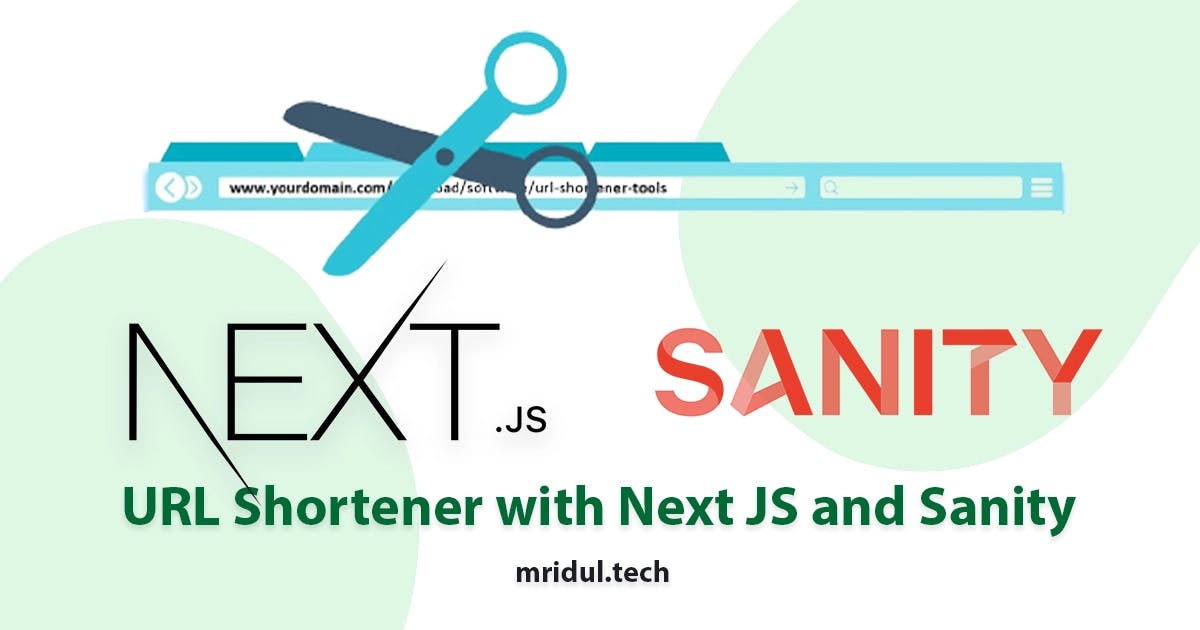
In today’s fast-paced digital world, URL shorteners have become essential tools for simplifying and sharing links. They provide concise and memorable URLs that are easier to manage and share across various platforms. If you’re looking to create your own URL shortener using Next JS and Sanity, you’re in the right place. This article will guide you through the process, providing detailed explanations and expert tips along the way.
Understanding URL Shorteners
URL shorteners are tools that transform long and complex URLs into shorter, more manageable links. These shortened links typically redirect users to the original URL when clicked. They are widely used in social media posts, email marketing campaigns, and any scenario where long URLs may be inconvenient or visually unappealing. By condensing URLs, shorteners improve user experience and make it easier to track and analyze link performance.
Introducing Next JS and Sanity
Next JS is a powerful React framework that enables server-side rendering (SSR) and static site generation (SSG) for web applications. It provides a filename routing system, allowing you to define your application’s routes and handle navigation seamlessly. Another notable feature of Next JS is its excellent integration with APIs, enabling easy data fetching from external sources or internal APIs. This makes it ideal for connecting with your Sanity backend and retrieving data for your URL shortener.
Sanity is a headless content management system (CMS) that allows you to structure and manage content with ease. It provides a flexible and customizable schema, allowing you to define your own data models and content types. This gives you full control over the structure and organization of your data, making it ideal for building a URL shortener where you need to manage URLs, redirect information, and related data.
Setting Up Your Development Environment
Before diving into building a URL shortener with Next JS and Sanity, you need to set up your development environment. Ensure that you have Node.js and npm (Node Package Manager) installed on your machine. You can easily install them by visiting the official Node.js website and following the instructions for your operating system.
You can find the Full code for this Project on GitHub
Creating a New Next JS Project
To create an URL Shortener with Next JS, you’ll need to create a new project. Open your terminal or command prompt and navigate to your desired project directory. Run the following command to generate a new Next JS project:
npx create-next-app shortener-frontend-nextjs
#or
yarn create-next-app shortener-frontend-nextjs
This command creates a new Next JS project named “shortener-frontend-nextjs” in the current directory.
The code for this project for the Frontend Part for Next JS is on GitHub
Also Read: How to use SCSS with Tailwind in Next JS
Installing and Configuring Sanity
Now that you have a Next JS project, it’s time to install and configure Sanity. Navigate to your project directory and run the following command:
npm install -g @sanity/cli
Now sanity is installed in your machine. So let’s create a new sanity project. To create a new sanity project run the command below.
sanity init --coupon modernfrontends22
This will create a sanity project with a boosted free plan, which includes –
- 200k API requests
- 1M API CDN requests
- 20GB Bandwidth.
Now follow the steps in the command line and create a sanity project with some example datasets. Here is an example of project creation
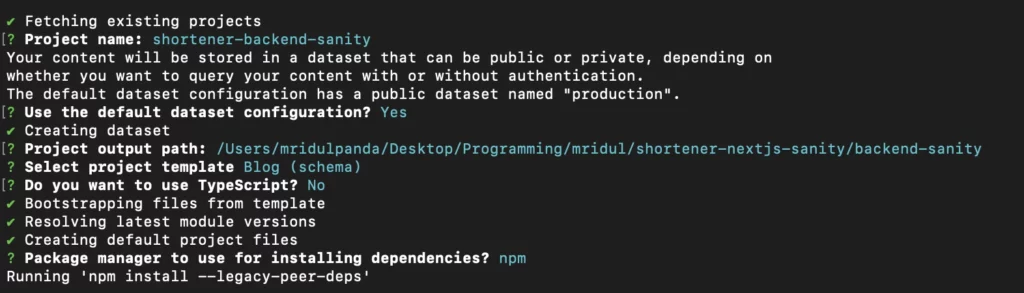
The code for this project for the Backend Part for Sanity is on GitHub
Building the Shortener Backend with Sanity
Sanity acts as the backend for your URL shortener, allowing you to manage and store URLs, redirect information, and other related data. You’ll define a schema in Sanity that reflects the structure of your data. Create a file named redirect.js
in the schema
folder. Here we will keep the src unique with isUnique: true
. Here’s an example schema for the URL shortener:
// schemas/redirect.js
export default {
name: "redirect",
title: "Redirect",
type: "document",
fields: [
{
name: "src",
title: "Source",
type: "string",
isUnique: true,
validation: (Rule) => Rule.required(),
},
{
name: "destination",
title: "Destination",
type: "string",
validation: (Rule) => Rule.required(),
},
],
preview: {
select: {
title: "src",
},
},
};
Now import that schema to the main schema. So that we can use that.
// schemas/redirect.js
import redirect from './redirect'
export const schemaTypes = [redirect]
Thats it. Our sanity setup is done. Now run the sanity server with npm run dev
command. The server will start at http://localhost:3333
. Now go to that URL and login with your account. You will see the redirect
content type. Now click on the pencil icon to create a new entry. After filling the details make sure to publish it.
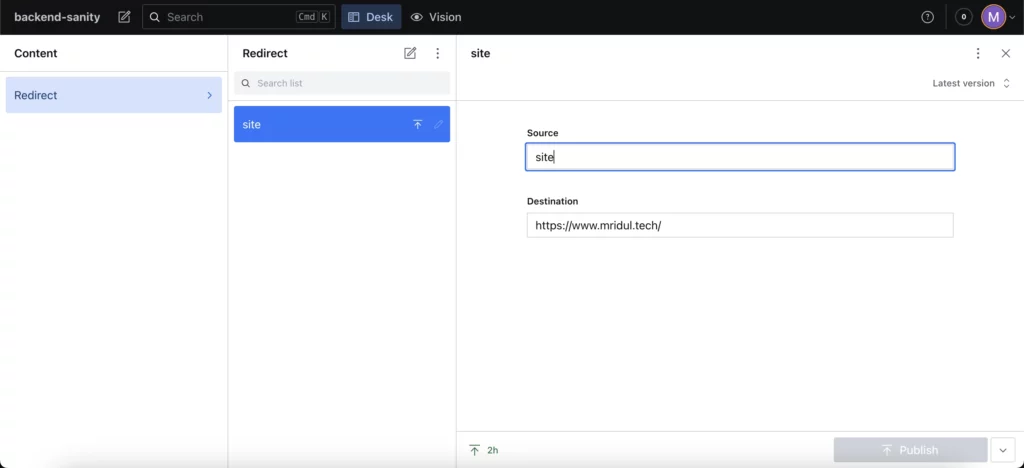
Also Read: Next JS Project Ideas to Boost Your Portfolio
Integrating Next JS with Sanity
Now that you have your Sanity backend set up, it’s time to integrate it with your Next JS frontend. Next JS provides excellent support for fetching data during server-side rendering (SSR) and static site generation (SSG). You’ll use Sanity’s JavaScript client library to interact with the Sanity API from your Next JS application.
To install the Sanity client library, run the following command in your Next JS project directory:
npm install @sanity/client
Now Create a Sanity client file and configure that with your sanity projectId
and creds. You will find the projectId
in your sanity repository in sanity.cli.js
and also in sanity.config.js
file. The project ID is important so keep it secret. Here is an example of sanity client in Next JS app –
import { createClient } from "@sanity/client";
const SanityClient = createClient({
projectId: "<project-id>",
dataset: "production",
useCdn: true,
apiVersion: "2022-01-12",
});
export default SanityClient;
Implementing Redirects
for URL Shortener with Next JS
When a user clicks on a shortened URL, your application needs to redirect them to the corresponding original URL. Here’s a basic example of a redirect handler:
// /pages/[alias].js
import { useRouter } from "next/router";
import { useEffect } from "react";
import SanityClient from "../src/client/sanity";
const AliasView = ({ error }) => {
const router = useRouter();
useEffect(() => {
if (error) {
router.push("/");
}
// eslint-disable-next-line
}, []);
return null;
};
export async function getServerSideProps({ params }) {
const alias = params.alias;
const url = await SanityClient.fetch(
`*[_type == "redirect" && src == "${alias}"][0]{
src,
destination,
}`
);
if (url && url?.src && url?.destination) {
return {
redirect: {
destination: url.destination,
permanent: false,
},
};
}
return {
props: {
error: "error",
},
};
}
export default AliasView;
Also Read: How to Use SVG in Next JS?
Deploying Your URL Shortener
Now we have finished the project, we can deploy our app. For Sanity, we can use the sanity deploy service. Run the command sanity deploy
and you need to choose your unique studio name and sanity will deploy the project.
For Next JS we can use vercel to deploy our project. Vercel is the creator of Next JS so it will be easy to deploy our Next JS Project at Vercel. For that create a Vercel account and connect it with your GitHub account and Just import the project and deploy. For a deploy step by step guide check How to Deploy Next JS app on Vercel.
Troubleshooting Common Issues for URL Shortener with Next JS
When working on your URL shortener project, you may encounter various challenges and issues. Here are some common problems and their potential solutions:
- URL Resolution: Ensure that your redirect logic correctly resolves shortened URLs to their corresponding original URLs.
- Sanity API Errors: Check the Sanity documentation and API reference for error codes and possible solutions.
- Deployment Errors: Review your deployment configuration and ensure that all required dependencies are installed correctly.
Conclusion
In this comprehensive guide, we explored the process of creating a URL shortener using Next JS and Sanity. From setting up your development environment to deploying your application, you’ve learned the essential steps and best practices for building a powerful and secure URL shortener. By following the outlined instructions and incorporating your own customizations, you can create a unique and effective tool for simplifying and managing URLs.
Remember, building a URL shortener requires a combination of technical expertise, attention to detail, and a user-centric mindset. Embrace the possibilities and unleash the potential of Next JS and Sanity in creating your own URL shortening solution.
You may also like
How to add Google Web Stories in Next JS

Dec 14, 2023
·10 Min Read
In the fast-paced digital world, user engagement is key to the success of any website. One effective way to captivate your audience is by incorporating Google Web Stories into your Next JS website. These visually appealing and interactive stories can make your content more engaging and shareable. In this comprehensive guide, we’ll walk you through […]
Read More
How to send Emails in Next JS for Free using Resend
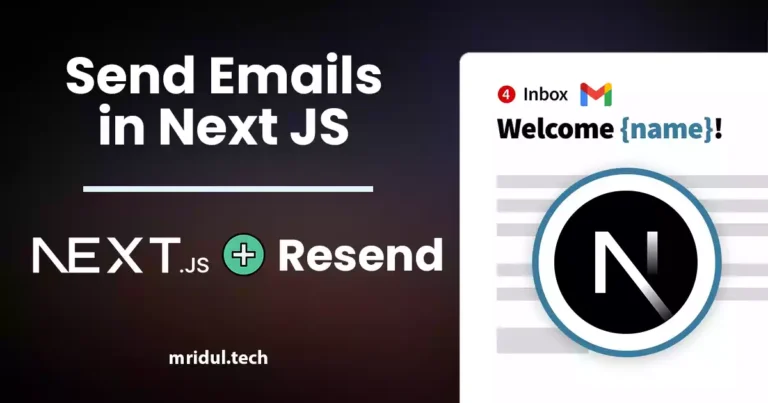
Nov 10, 2023
·7 Min Read
Sending emails in web applications is a crucial feature, and in this article, we will explore how to send Emails in Next JS for free using Resend. Next JS is a popular framework for building React applications, and Resend is a handy tool for email integration. By the end of this guide, you’ll have the […]
Read More
How to add Google Login in Next.js with Appwrite

Nov 01, 2023
·7 Min Read
Are you looking to enhance user authentication in your Next.js application? Integrating Social Login with Appwrite can be a game-changer. Add Google Login to your Next.js app with Appwrite. This article will guide you through the process, and practical tips to add Google Login in Next.js with Appwrite. GitHub Code: Google Login in Next.js with […]
Read More
JavaScript Project Ideas to Boost Your Portfolio

Oct 13, 2023
·3 Min Read
JavaScript is the backbone of web development, and mastering it is essential for any aspiring developer. While learning the basics is crucial, building real-world projects is the key to solidifying your knowledge. In this comprehensive guide, we’ll present a diverse range of JavaScript project ideas that cater to different skill levels and interests. These projects […]
Read More
How to Generate robots.txt in Next JS?

Oct 05, 2023
·4 Min Read
In the world of web development and search engine optimization (SEO), ensuring that your website is easily accessible and properly indexed by search engines is paramount. One essential tool that aids in this process is the creation of a robots.txt file. In this comprehensive guide, we, the experts, will walk you through the process of […]
Read More
How to Generate Sitemap in Next JS?
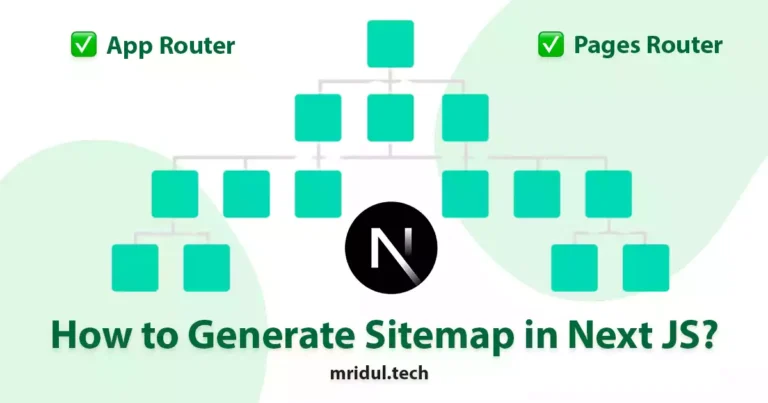
Sep 28, 2023
·8 Min Read
In today’s digital landscape, optimizing your website’s SEO is crucial to attracting more organic traffic and ranking higher on search engine result pages (SERPs). One essential aspect of SEO is creating a sitemap, which helps search engines crawl and index your website efficiently. If you’re using Next JS for your website development, this guide will […]
Read More