How to add Styled components in Next.js App router

Styled components have become a popular choice for styling React applications due to their simplicity and flexibility. When it comes to integrating styled components into a Next.js application, it’s essential to understand how to leverage them effectively within the app router. In this guide, we’ll explore step-by-step how to add styled components to a Next.js app router to enhance the visual appeal and maintainability of your application.
What is Styled Components?
Styled components are a CSS-in-JS library that allows developers to write CSS directly within their JavaScript code. They offer a more cohesive approach to styling by encapsulating styles within individual components, making it easier to manage and reuse styling logic across the application.
Also Read: How to use CSS Animations with Styled Components
Setting Up Next.js App
Before we can start integrating styled components, we need to set up a Next.js project. If you haven’t already installed Next.js, you can do so by running the following command:
npx create-next-app my-next-app
This command will create a new Next.js project with all the necessary dependencies installed.
Styled Components in Next.js
Installing Styled Components in Next.js
To begin using styled components in our Next.js app, we need to install the styled-components
package. We can do this by running the following command:
npm install styled-components
#or
yarn add styled-components
Setup Styled Components in Next.js App Router
First, we need to enable the styledComponents
compiler in the next.config.js
.
/** @type {import('next').NextConfig} */
const nextConfig = {
compiler: {
styledComponents: true,
},
};
export default nextConfig;
Add the Global style registry
A global style registry component that gathers all the styles and applies them to the tag is suggested to be implemented by Next.js. Make sure you have the lib/registry.tsx
file created and add the following:
'use client'
import React, { useState } from 'react'
import { useServerInsertedHTML } from 'next/navigation'
import { ServerStyleSheet, StyleSheetManager } from 'styled-components'
export default function StyledComponentsRegistry({
children,
}: {
children: React.ReactNode
}) {
// Only create stylesheet once with lazy initial state
// x-ref: https://reactjs.org/docs/hooks-reference.html#lazy-initial-state
const [styledComponentsStyleSheet] = useState(() => new ServerStyleSheet())
useServerInsertedHTML(() => {
const styles = styledComponentsStyleSheet.getStyleElement()
styledComponentsStyleSheet.instance.clearTag()
return <>{styles}</>
})
if (typeof window !== 'undefined') return <>{children}</>
return (
<StyleSheetManager sheet={styledComponentsStyleSheet.instance}>
{children}
</StyleSheetManager>
)
}
Import StyledComponentsRegistry
into your root layout.tsx
file, then wrap children in it:
import StyledComponentsRegistry from './lib/registry'
export default function RootLayout(props: React.PropsWithChildren) {
return (
<html>
<body>
<StyledComponentsRegistry>
{props.children}
</StyledComponentsRegistry>
</body>
</html>
)
}
Also Read: How to use both Tailwind and Styled Components in Next JS
Add Styled Components global styles
You can add typefaces, reset or normalise CSS, and apply any other style you want to use worldwide in the global styles section.
Using the createGlobalStyle()
function, you can accomplish this. Make the file styles/GlobalStyles.ts
and add the following to it:
import { createGlobalStyle } from 'styled-components';
const GlobalStyles = createGlobalStyle`
// your global styles
`;
export default GlobalStyles;
Please import and add the GlobalStyles component to your root layout.tsx
file.
import StyledComponentsRegistry from './lib/registry'
import GlobalStyles from './styles/GlobalStyles';
export default function RootLayout(props: React.PropsWithChildren) {
return (
<html>
<body>
<StyledComponentsRegistry>
<GlobalStyles />
{props.children}
</StyledComponentsRegistry>
</body>
</html>
)
}
Also Read: How to use both Tailwind and Styled Components in Next JS
Add a theme in Styled Components
It’s possible that you need to access global variables in several components, such as font sizes, colours, or other configurations. To do that, you can utilise a theme:
Make a file called styles/theme.ts
and fill it with your colour scheme:
const theme = {
colors: {
colorName1: '#001100',
colorName2: '#001100',
...
},
};
export default theme;
Import ThemeProvider
and your theme into your root layout.tsx file, then wrap children in it:
'use client';
import StyledComponentsRegistry from './lib/registry'
import GlobalStyles from './styles/GlobalStyles';
import { ThemeProvider } from 'styled-components';
import theme from './styles/theme';
export default function RootLayout(props: React.PropsWithChildren) {
return (
<html>
<body>
<StyledComponentsRegistry>
<GlobalStyles />
<ThemeProvider theme={theme}>
{props.children}
</ThemeProvider>
</StyledComponentsRegistry>
</body>
</html>
)
}
Access the theme
in your components:
import { styled } from 'styled-components';
export const MyDiv = styled.div`
background-color: ${({ theme }) => theme.colors.colorName1};
`;
Conclusion
In conclusion, adding styled components to a Next js app router can enhance the visual appeal and maintainability of your application. By following the steps outlined in this guide and adhering to best practices, you can leverage the power of styled components to create beautiful and performant Next.js applications.
You may also like
How to add Google Web Stories in Next JS

Dec 14, 2023
·10 Min Read
In the fast-paced digital world, user engagement is key to the success of any website. One effective way to captivate your audience is by incorporating Google Web Stories into your Next JS website. These visually appealing and interactive stories can make your content more engaging and shareable. In this comprehensive guide, we’ll walk you through […]
Read More
How to send Emails in Next JS for Free using Resend
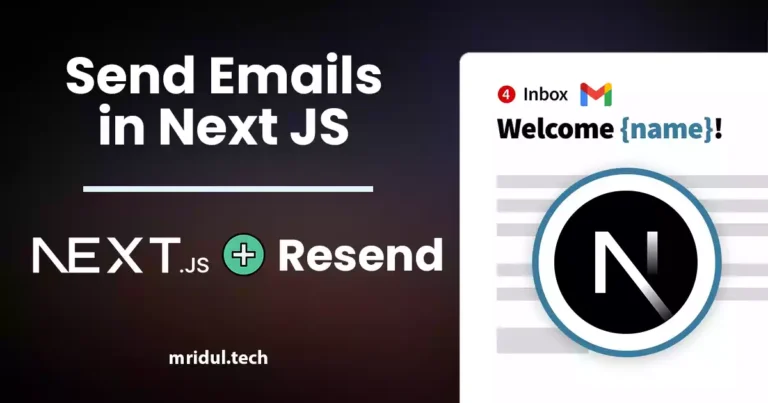
Nov 10, 2023
·7 Min Read
Sending emails in web applications is a crucial feature, and in this article, we will explore how to send Emails in Next JS for free using Resend. Next JS is a popular framework for building React applications, and Resend is a handy tool for email integration. By the end of this guide, you’ll have the […]
Read More
How to add Google Login in Next.js with Appwrite

Nov 01, 2023
·7 Min Read
Are you looking to enhance user authentication in your Next.js application? Integrating Social Login with Appwrite can be a game-changer. Add Google Login to your Next.js app with Appwrite. This article will guide you through the process, and practical tips to add Google Login in Next.js with Appwrite. GitHub Code: Google Login in Next.js with […]
Read More
How to add Protected Routes in Next JS

Oct 28, 2023
·5 Min Read
In the world of web development, security is paramount. Whether you are building a simple blog or a complex web application, protecting certain routes and pages from unauthorized access is a crucial step. In this comprehensive guide, we will walk you through the process of adding protected routes in Next JS, ensuring that your web […]
Read More
How to run localhost 3000 on https in Next JS

Oct 20, 2023
·2 Min Read
In the ever-evolving world of web development, having a secure local development environment is crucial. If you’re working with Next.js and need to run localhost on HTTPS with port 3000, you’re in the right place. In this comprehensive guide, we will walk you through the process step by step, ensuring you have a secure and […]
Read More
How to Generate robots.txt in Next JS?

Oct 05, 2023
·4 Min Read
In the world of web development and search engine optimization (SEO), ensuring that your website is easily accessible and properly indexed by search engines is paramount. One essential tool that aids in this process is the creation of a robots.txt file. In this comprehensive guide, we, the experts, will walk you through the process of […]
Read More