How to use CSS Animations with Styled Components
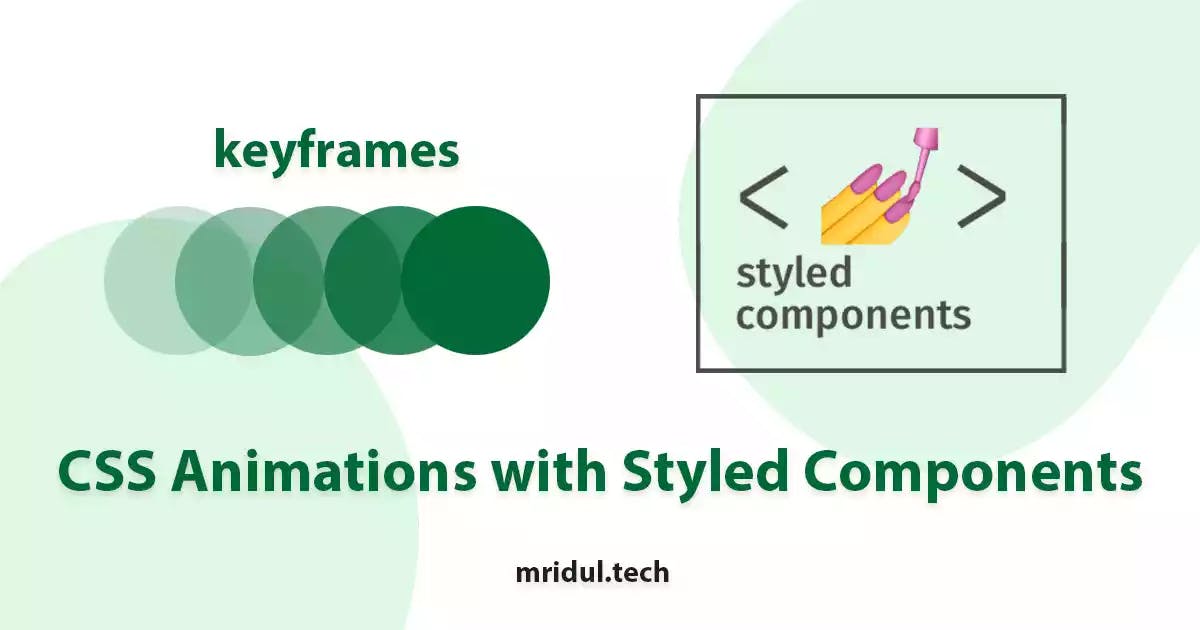
The styled component is one of the most popular libraries for giving styles to React JS and Next JS projects. It has over 4.9 Million downloads in NPM. With styled-components, we can write the CSS in the component file, providing so much maintainable and clean code. But when you try to add CSS keyframes
the way is not straightforward. So in this article, we will see ways to add CSS Animations with Styled Components with keyframes.
CSS animations with keyframes
In vanilla CSS we use keyframes to handle the complex animation in the elements. In this example, we are going to animate a circle with the CSS keyframes and later we are going to do it with styled components.
// html
<div class="circle></div>
// css
.circle {
width: 100px;
height: 100px;
border-radius: 50%;
background-color: red;
animation: rotateslide 5s linear infinite;
}
The animation for the div will be a slide animation. So here is the keyframe for the animation.
@keyframes rotateslide {
0% {
transform: translate(0, 0);
}
25% {
transform: translate(100px, 0);
}
50% {
transform: translate(100px, 100px);
}
75% {
transform: translate(0, 100px);
}
100% {
transform: translate(0, 0);
}
}
Also Read: How to add Google AdSense in Next JS
CSS Animations in Styled Components
But how we are gonna add that keyframe in a styled-components? We are going to see two ways where we will add the keyframes.
Keyframes from styled-components
This is the best way to add keyframes in styled-components. With this method, you can reuse your keyframes in any styled component animation, So there will be clean code and reusability.
1. Styled Components has built-in keyframes support. We can import that and define our keyframe animation in it.
import { keyframes } from 'styled-components';
2. Now we can use that keyframes
like a normal styled component. So here is our keyframe component.
const rotateslide = keyframes`
0% {
transform: translate(0, 0);
}
25% {
transform: translate(100px, 0);
}
50% {
transform: translate(100px, 100px);
}
75% {
transform: translate(0, 100px);
}
100% {
transform: translate(0, 0);
}
`;
3. Now we can use the component in any animation style. But the syntax is a bit different from the normal CSS animation. Look at the difference between CSS animation and styled component animation. In the styled-components animation, we need to add the animation name with string interpolation.
// css animation
animation: rotateslide 5s linear infinite;
// styled-components animation
animation: ${rotateslide} 5s linear infinite;
Also Read: How to use both Tailwind and Styled Components in Next JS
4. But remember one thing, Define the keyframe component before using it. Otherwise, you’ll get the undefined
error. Let’s look at the complete code –
import React from 'react';
import styled, { keyframes } from 'styled-components';
const KeyframeAnimation = () => {
return <Container></Container>;
};
export default KeyframeAnimation;
const rotateslide = keyframes`
0% {
transform: translate(0, 0);
}
25% {
transform: translate(100px, 0);
}
50% {
transform: translate(100px, 100px);
}
75% {
transform: translate(0, 100px);
}
100% {
transform: translate(0, 0);
}
`;
const Container = styled.div`
margin: 10px;
width: 100px;
height: 100px;
border-radius: 50%;
background-color: red;
animation: ${rotateslide} 5s linear infinite;
`;
Also Read: Next JS Project Ideas to Boost Your Portfolio
CSS keyframes in Styled Components
With this method, you can use the keyframes like CSS keyframes animation. But there is a problem with it. You have to add the keyframe on the styled component you want to use. So for every animation, you need to add the keyframes.
1. In this method we can add the keyframes after our styled component styles. And the animation syntaxes will be the same as CSS. Let’s look at one example –
import React from 'react';
import styled from 'styled-components';
const KeyframeAnimation = () => {
return <Container></Container>;
};
export default KeyframeAnimation;
const Container = styled.div`
margin: 10px;
width: 100px;
height: 100px;
border-radius: 50%;
background-color: red;
animation: rotateslide 5s linear infinite;
@keyframes rotateslide {
0% {
transform: translate(0, 0);
}
25% {
transform: translate(100px, 0);
}
50% {
transform: translate(100px, 100px);
}
75% {
transform: translate(0, 100px);
}
100% {
transform: translate(0, 0);
}
}
`;
Now, look at the code above. We have defined the keyframe in that styled component under our styles.
And this is it. In the above two ways, we can add CSS Animations with Styled Components in our React JS or Next JS Project.
You may also like
Top 5 CSS Libraries for Web Developers in 2023
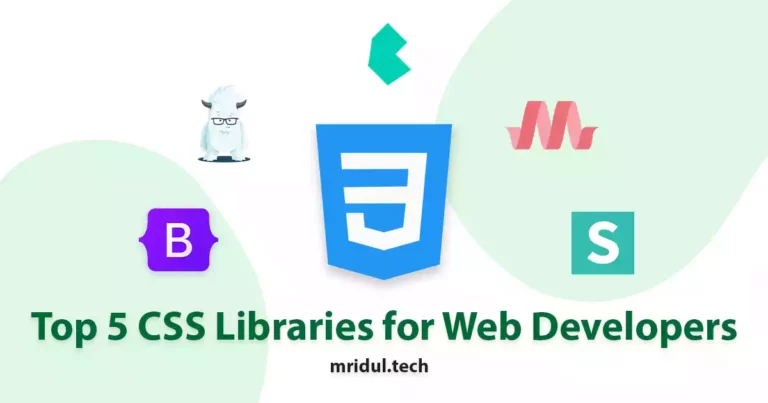
Mar 30, 2023
·6 Min Read
CSS or Cascading Style Sheets is an essential part of modern web development. It enables developers to create visually appealing and responsive websites that engage users. However, developing CSS from scratch can be time-consuming and challenging. Fortunately, there are numerous CSS libraries available that make CSS development more manageable, efficient, and effective. In this article, […]
Read More
How to Create Heartbeat Animation in CSS
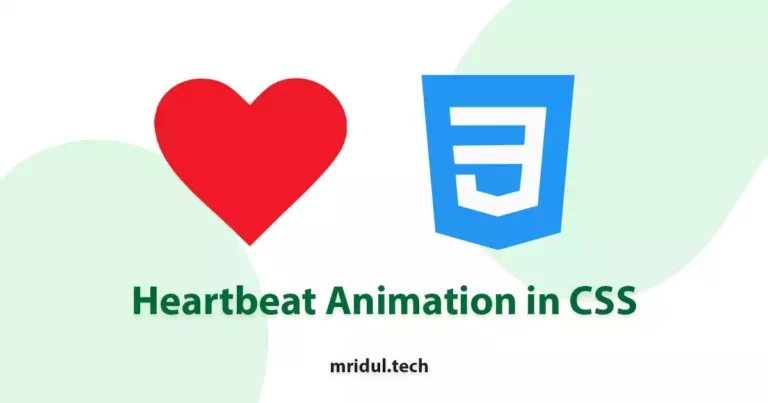
Feb 03, 2023
·3 Min Read
With only HTML and CSS you can create a lot of create animation and a lot of shapes in the web. And in this article you will learn how to draw a heart shape and add Heartbeat Animation in CSS to the shape Get the full source code of the heartbeat animation on GitHub Draw […]
Read More