How to Highlight Code Syntax in Next JS
Mridul Panda
Jan 15, 2023
·4 Min Read
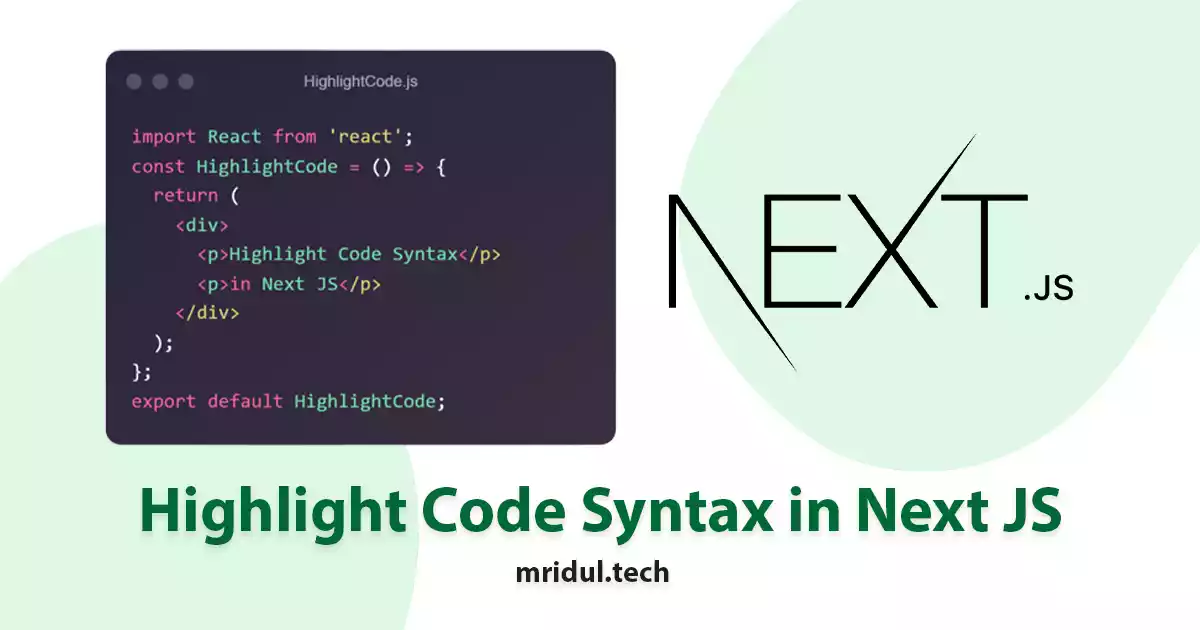
If you have a blog or a landing page that has code snippets then chances are high that you want to highlight them with colours, just like we see in a code editor. The code highlight can be done manually with different classes but it is hard to track and add many classenames
. And if you are using Next JS then you need it to be server-side rendered, which is a headache for any website owner or developer. That’s why in this article we are going to highlight Code Syntax in Next JS app without any headache.
Install Highlight.js for Highlight Code Syntax
For code highlighting, we are going to use an npm package named Highlight.js
. This is a very popular library with over 7.3 Million weekly downloads. Highlight js has both server and browser support. We can use this in any markup and in any framework. Highlight js has both CDN support and NPM support but we are going to use the npm version for the Next js. Let’s install the package –
npm install highlight.js
or
yarn add highlight.js
In the main library of Highlight.js, more than 180 languages are supported. To accommodate even more languages, there are 3rd party language definitions available. But we will be using only the javascript highlighting here. You can find all the supported languages in the Highlight.js Repository.
// import Highlight.js and the languages you need
import hljs from 'highlight.js/lib/core';
import javascript from 'highlight.js/lib/languages/javascript';
hljs.registerLanguage('javascript', javascript)
Also Read: How to add Google AdSense in Next JS
Highlight Code Syntax in Next JS
For the highlight.js to run we need to initialize it in a useEffect
and need to run the function hljs.highlightAll()
after that highlight.js
will add code highlights in the code. You can render your code snipped in dangerouslySetInnerHTML
if you are using richtext
. Let’s look at the code.
import React, { useEffect } from 'react';
const HigligtCode = ({ content }) => {
useEffect(() => {
hljs.highlightAll();
}, []);
return (
<div>
<div
dangerouslySetInnerHTML={{
__html: content,
}}
/>
</div>
);
};
Also Read: How to Build PWA with Next JS?
Alternatively, if you are having simple code that you want to highlight you can use hljs.highlight()
function. Let’s see an example of that –
const HigligtCode = ({ content }) => {
const code = "console.log('Highlighted Code')"
const highlightedCode = hljs.highlight(code , { language: 'javascript' }).value
return (
<div>
<div
dangerouslySetInnerHTML={{
__html: highlightedCode ,
}}
/>
</div>
);
};
The hljs.highlight()
generates an HTML string for you. This is different from the hljs.highlightAll()
, which scans the page on the client side and updates it with syntax highlighting. Since hljs.highlightAll()
runs client-side, it can unintentionally spill out into other parts of your page and apply highlighting where you don’t want it.
Themes to Highlight Code Syntax
Highlight.js
provides so many themes both in dark mode and light mode. You can simply import a theme CSS file from the package and it will be applied to the snippet. Example of theme import –
import hljs from 'highlight.js/lib/core';
import javascript from 'highlight.js/lib/languages/javascript';
// Import a theme from the package
import 'highlight.js/styles/github-dark.css';
Also Read: Next JS Project Ideas to Boost Your Portfolio
Let’s look at all the themes provided by the highlight.js package –
- ally-darkcess
- a1fy-light.css
- agate.css
- androidstudio.css
- an-old-hope.css
- arduino-light.css
- arta.css
- ascetic.css
- atom-one-dark.css
- atom-one-dark-reasonable.css
- atom-one-light.css brown-paper.css
- codepen-embed.css
- color-brewer.css
- dark.css
- default.css
- devibeans.css docco.css
- far.css
- felipec.css
- foundation.css
- github.css
- github-dark.css
- github-dark-dimmed.css
- gml.css
- googlecode.css
- gradient-dark.css
- gradient-light.css
- grayscale.css
- hybrid.css
- idea.css
- intelli-light.css
- ir-black.css
- isbl-editor-dark.css
- isbl-editor-light.css
- kimbie-dark.css
- kimbie-light.css
- lightfair.css
- lioshi.css
- magula.css
- mono-blue.css
- monokai.css
- monokai-sublime.css
- night-owl.css
- nnfx-dark.css
- nnfx-light.css
- nord.css
- obsidian.css
- panda-syntax-dark.css
- panda-syntax-light.css
- paraiso-dark.css
- paraiso-light.css
- pojoaque.css
- purebasic.css
- qtcreator-dark.css
- qtcreator-light.css
- rainbow.css
- routeros.css
- school-book.css
- shades-of-purple.css
- srcery.css
- stackoverflow-dark.css
- stackoverflow-light.css
- sunburst.css
- tokyo-night-light.css
- tomorrow-night-blue.css
- tomorrow-night-bright.css
- vs.css
- vs2015.css
- xcode.css
- xt256.css
That’s it for the tutorial hope you will find this helpful.
You may also like
How to add Styled components in Next.js App router
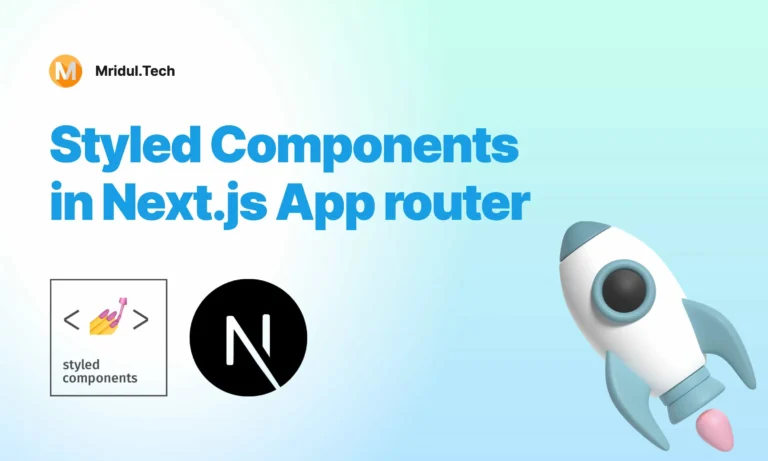
May 11, 2024
·4 Min Read
Styled components have become a popular choice for styling React applications due to their simplicity and flexibility. When it comes to integrating styled components into a Next.js application, it’s essential to understand how to leverage them effectively within the app router. In this guide, we’ll explore step-by-step how to add styled components to a Next.js […]
Read More
How to add Google Web Stories in Next JS
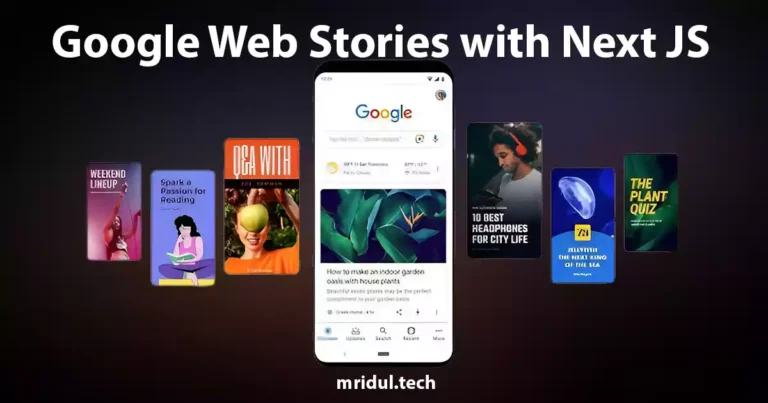
Dec 14, 2023
·10 Min Read
In the fast-paced digital world, user engagement is key to the success of any website. One effective way to captivate your audience is by incorporating Google Web Stories into your Next JS website. These visually appealing and interactive stories can make your content more engaging and shareable. In this comprehensive guide, we’ll walk you through […]
Read More
How to send Emails in Next JS for Free using Resend
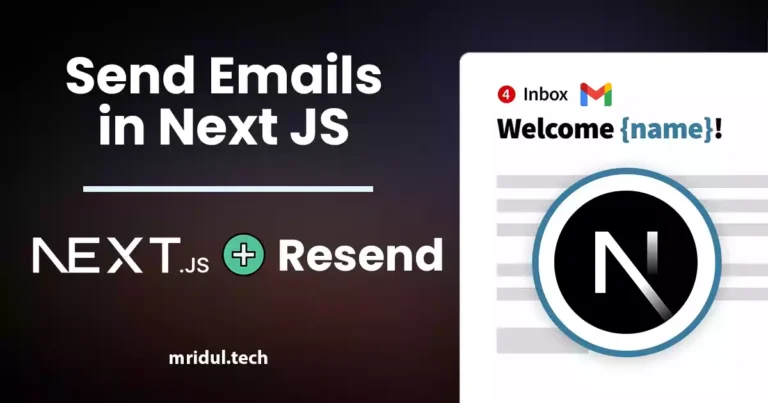
Nov 10, 2023
·7 Min Read
Sending emails in web applications is a crucial feature, and in this article, we will explore how to send Emails in Next JS for free using Resend. Next JS is a popular framework for building React applications, and Resend is a handy tool for email integration. By the end of this guide, you’ll have the […]
Read More
How to add Google Login in Next.js with Appwrite
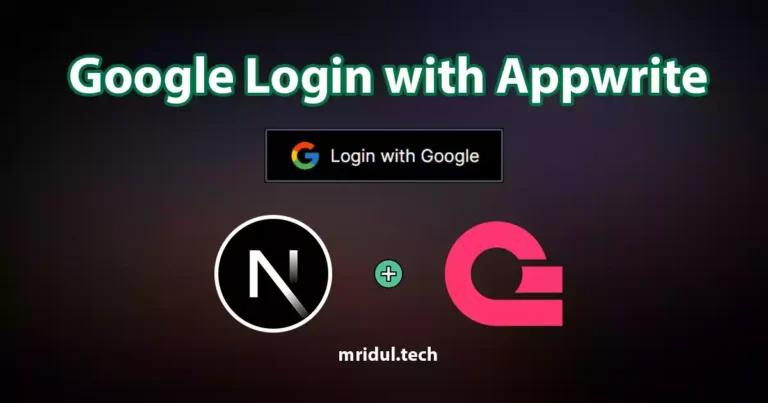
Nov 01, 2023
·7 Min Read
Are you looking to enhance user authentication in your Next.js application? Integrating Social Login with Appwrite can be a game-changer. Add Google Login to your Next.js app with Appwrite. This article will guide you through the process, and practical tips to add Google Login in Next.js with Appwrite. GitHub Code: Google Login in Next.js with […]
Read More
How to add Protected Routes in Next JS
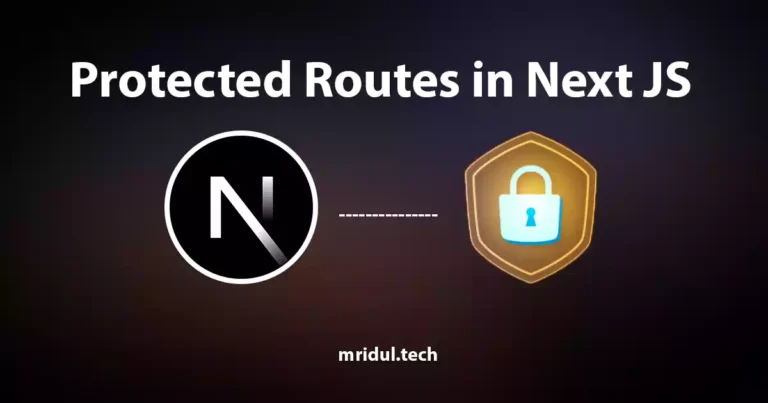
Oct 28, 2023
·5 Min Read
In the world of web development, security is paramount. Whether you are building a simple blog or a complex web application, protecting certain routes and pages from unauthorized access is a crucial step. In this comprehensive guide, we will walk you through the process of adding protected routes in Next JS, ensuring that your web […]
Read More
How to run localhost 3000 on https in Next JS
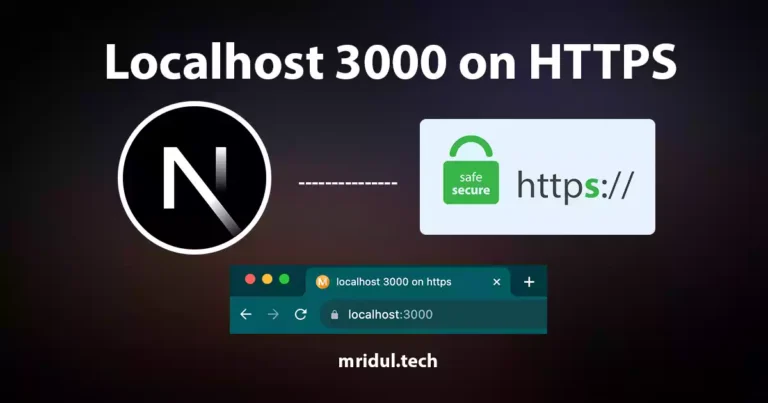
Oct 20, 2023
·2 Min Read
In the ever-evolving world of web development, having a secure local development environment is crucial. If you’re working with Next.js and need to run localhost on HTTPS with port 3000, you’re in the right place. In this comprehensive guide, we will walk you through the process step by step, ensuring you have a secure and […]
Read More