React Hook useEffect Has a Missing Dependency: How to Fix It
Mridul Panda
Mar 10, 2023
·6 Min Read
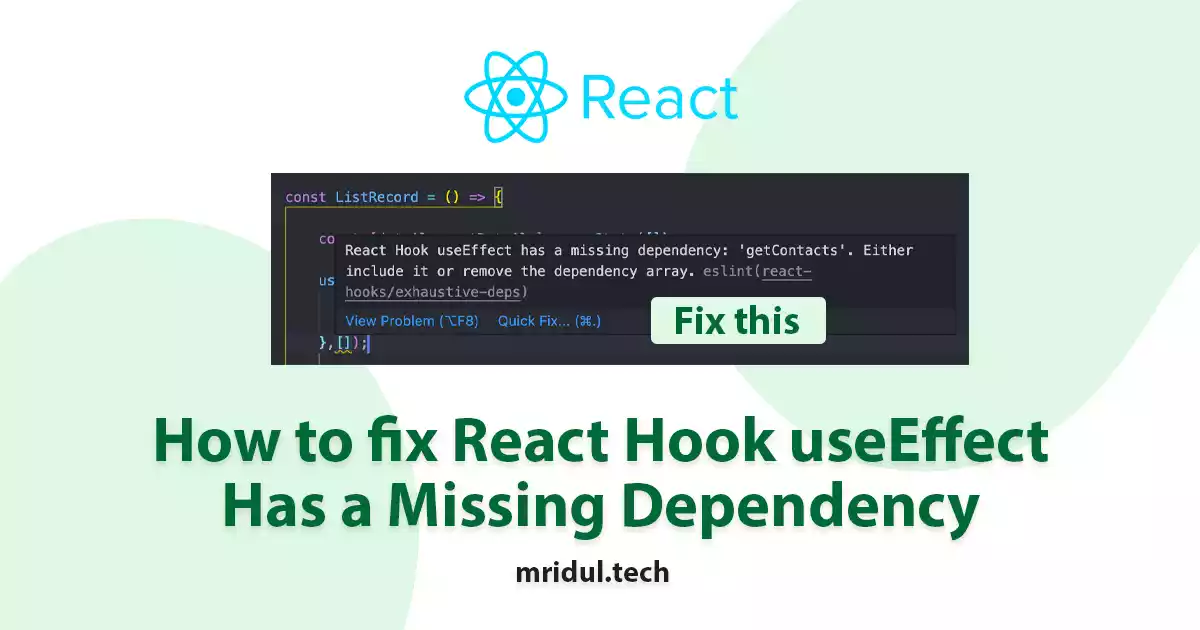
React is one of the most popular JavaScript libraries used for building user interfaces. One of the main advantages of React is the use of hooks, which allow you to use state and other React features in functional components. One of the most commonly used hooks is useEffect, which is used for handling side effects like fetching data, setting up subscriptions, and more. However, one common error that developers encounter is the “React hook useEffect has a missing dependency” warning. In this article, we’ll explain what this warning means, why it occurs, and how to fix it.
What is useEffect Hook?
The useEffect hook is a function that lets you run side effects in functional components. A side effect is any operation that affects something outside of the component. This can include fetching data from an API, updating the DOM, or setting up subscriptions.
What is a Dependency in useEffect?
A dependency in useEffect is any value that is used inside the hook. The purpose of dependencies is to tell React when to run the hook. If a dependency changes, React will re-run the hook. This allows you to control when side effects occur.
What is the React Hook useEffect Has a Missing Dependency Warning?
The “React Hook useEffect has a Missing Dependency” warning is a warning that is displayed in the console when React detects that a dependency is missing from the useEffect hook. This means that the hook is using a value that is not listed as a dependency. If this warning is ignored, it can lead to bugs and unexpected behaviour in your application.
Why Does the Warning Occur?
The warning occurs when a value that is used inside the useEffect hook is not listed as a dependency. This can happen when a variable or function is used inside the hook but is not listed in the dependency array. When this happens, React does not know when to re-run the hook, which can lead to bugs and unexpected behaviour.
Also Read: 10 React Project Ideas to Boost Your Portfolio
How to Fix the React Hook useEffect Has a Missing Dependency Warning?
To fix the “React Hook useEffect has a Missing Dependency” warning, you need to add the missing dependency to the dependency array. The dependency array is the second argument to the useEffect hook. And it lists all the values that the hook depends on. By adding the missing dependency to the array, React will know when to re-run the hook.
Example 1: Adding Missing Dependency
Let’s say we have a simple component that fetches data from an API when it is mounted:
import React, { useState, useEffect } from 'react';
import axios from 'axios';
const MyComponent = () => {
const [data, setData] = useState(null);
useEffect(() => {
axios.get('/api/data')
.then((response) => {
setData(response.data);
})
.catch((error) => {
console.error(error);
});
}, []);
In this example, we are fetching data from the API using the Axios library. We are using the useEffect hook to run the code when the component is mounted, which is indicated by the empty dependency array. However, the warning useEffect has a Missing Dependency: Axios is displayed in the console.
To fix this warning, we need to add the Axios library to the dependency array:
import React, { useState, useEffect } from 'react';
import axios from 'axios';
const MyComponent = () => {
const [data, setData] = useState(null);
useEffect(() => {
axios.get('/api/data')
.then((response) => {
setData(response.data);
})
.catch((error) => {
console.error(error);
});
}, [axios]);
// rest of the component code
}
By adding axios
to the dependency array, we are telling React that the hook depends on this library, and it should re-run the hook if axios
changes.
Also Read: Next JS vs React: Which One Should You Choose?
Example 2: Using useCallback to Memoize Functions
In some cases, you may encounter the Missing Dependency warning when using functions inside the useEffect hook. For example:
import React, { useState, useEffect } from 'react';
const MyComponent = () => {
const [count, setCount] = useState(0);
const handleClick = () => {
setCount(count + 1);
};
useEffect(() => {
const intervalId = setInterval(handleClick, 1000);
return () => {
clearInterval(intervalId);
};
}, []);
// rest of the component code
}
In this example, we are using the handleClick
function inside the useEffect hook to increment the count
state every second. However, we are not listing handleClick
as a dependency, which causes the warning “React Hook useEffect has a Missing Dependency: ‘handleClick'” to be displayed in the console.
To fix this warning, we can use the useCallback
hook to memoize the function:
import React, { useState, useEffect, useCallback } from 'react';
const MyComponent = () => {
const [count, setCount] = useState(0);
const handleClick = useCallback(() => {
setCount(count + 1);
}, [count]);
useEffect(() => {
const intervalId = setInterval(handleClick, 1000);
return () => {
clearInterval(intervalId);
};
}, [handleClick]);
// rest of the component code
}
By using the useCallback
hook, we are memoizing the handleClick
function, which means that it will only be re-created when count
changes. We can then add handleClick
to the dependency array of the useEffect hook, which tells React to re-run the hook when handleClick
changes.
Also Read: How to use GitHub GraphQL API in React or Next JS
Best Practices for Using useEffect to avoid React Hook useEffect Has a Missing Dependency Warning
To avoid the useEffect has a Missing Dependency warning and ensure that your code runs as expected, here are some best practices for using the useEffect hook:
- Always list all dependencies in the dependency array, even if they are not used inside the hook.
- Use the
useCallback
hook to memoize functions that are used inside the hook. - If you need to access props inside the hook, pass them as dependencies to the hook.
- If you need to perform a side effect only once when the component is mounted, use an empty dependency array.
- If you need to perform a side effect whenever a certain value changes, add that value to the dependency array.
Conclusion
The useEffect
hook is a powerful tool for managing side effects in React components. However, it is important to understand how to use it correctly to avoid the “React Hook useEffect has a Missing Dependency” warning and ensure that your code runs as expected.
By following best practices and listing all dependencies in the dependency array, using the useCallback hook to memoize functions, and passing props as dependencies when needed, you can write clean and efficient code that works as intended. Remember to always pay attention to the warnings and errors in your console, as they can often help you identify and fix issues in your code.
A. The “React Hook useEffect has a Missing Dependency” warning is caused when the useEffect hook depends on a value that is not listed in the dependency array. This can happen when using variables, functions, or libraries inside the hook without listing them as dependencies.
A. Ignoring the “React Hook useEffect has a Missing Dependency” warning can lead to bugs and unexpected behaviour in your code. When a value is not listed in the dependency array, the hook may not re-run when that value changes, which can cause your code to become out of sync with the application state.
Listing too many dependencies in the dependency array can cause performance issues, as the hook will re-run whenever any of the listed dependencies change. To avoid this, only list the values that the hook depends on, and use the useCallback hook to memoize functions that are used inside the hook.
You may also like
React Hooks Cheatsheet
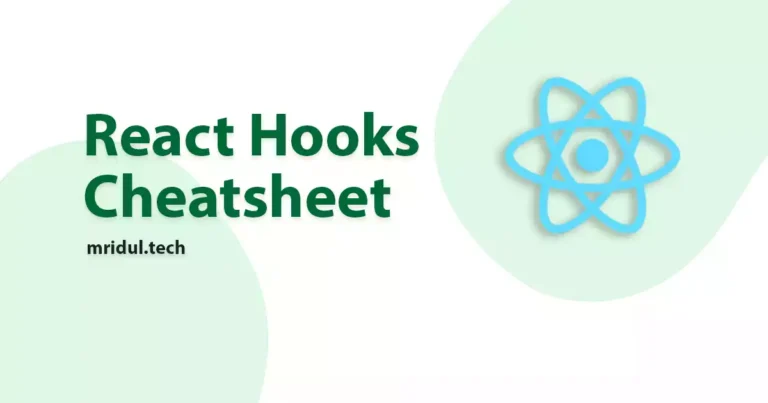
Jan 21, 2024
·4 Min Read
React, a popular JavaScript library for building user interfaces, introduced Hooks to make functional components more powerful and expressive. Let’s dive into the React Hooks Cheatsheet to understand how they enhance the development experience. React Hooks Cheatsheet In the ever-evolving landscape of web development, React Hooks have become indispensable for building dynamic and efficient user […]
Read More
React Emoji Picker: Add Emoji Picker in a React App
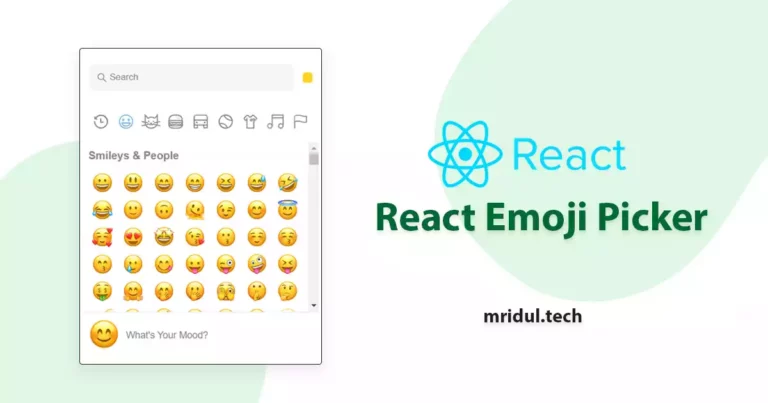
May 18, 2023
·3 Min Read
Emojis have become a staple in modern communication, with people using them to convey emotions and ideas in various contexts. As such, it’s important for developers to provide users with an easy and intuitive way to input emojis into their applications. This is where the React Emoji Picker comes in handy. This tool simplifies the […]
Read More
Creating a Stunning Image Gallery using React Image Gallery
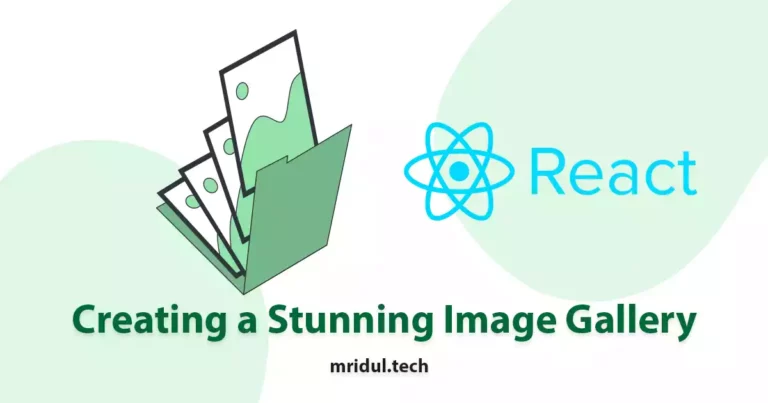
Apr 19, 2023
·5 Min Read
When it comes to building a website, a visually appealing image gallery can make a huge difference in the overall user experience. React Image Gallery is a popular and flexible Reactjs component library that can help you create a stunning gallery for your website. In this article, we will explore the features of React Image […]
Read More
Top 5 Platforms to Host React Apps for Free
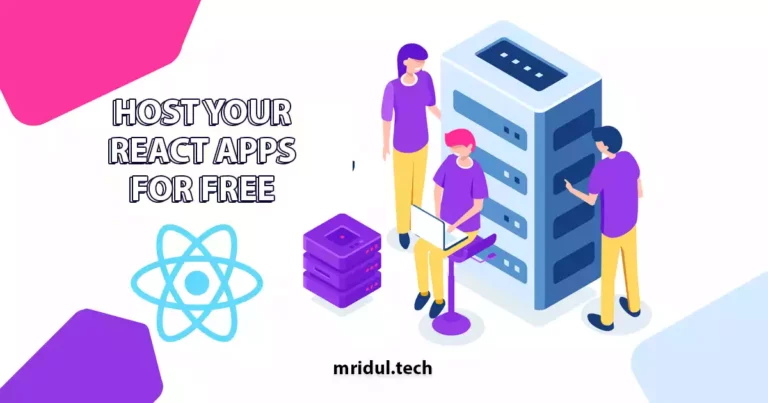
Apr 11, 2023
·7 Min Read
Learn about the best platforms to host your React apps for free. This guide will help you choose the right platform that suits your needs.
Read More
How to Upload Images to Cloudinary With React JS
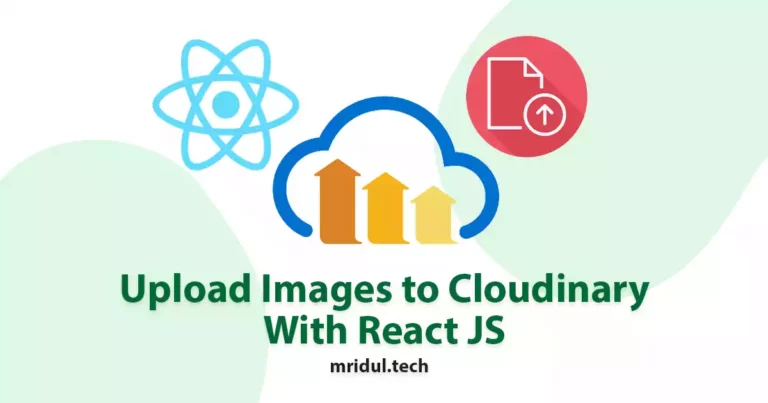
Apr 05, 2023
·6 Min Read
Are you struggling to upload images in your React JS application? Are you tired of the tedious process of configuring file uploads and handling image storage? If so, you are not alone. Many developers find image management in web applications to be a challenge. Fortunately, Cloudinary offers an easy solution. In this article, we will […]
Read More
How to Create a React Search Bar for Your Website
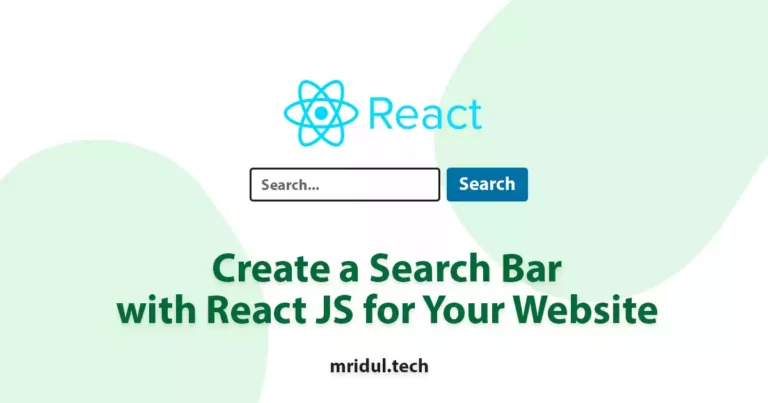
Mar 18, 2023
·6 Min Read
A search bar is an essential component of any website, allowing visitors to find the information they need quickly. If you are building a website using React, you can easily create a search bar to improve the user experience. In this article, we will guide you through the process of creating a React search bar […]
Read More