How to Create a React Search Bar for Your Website
Mridul Panda
Mar 18, 2023
·6 Min Read
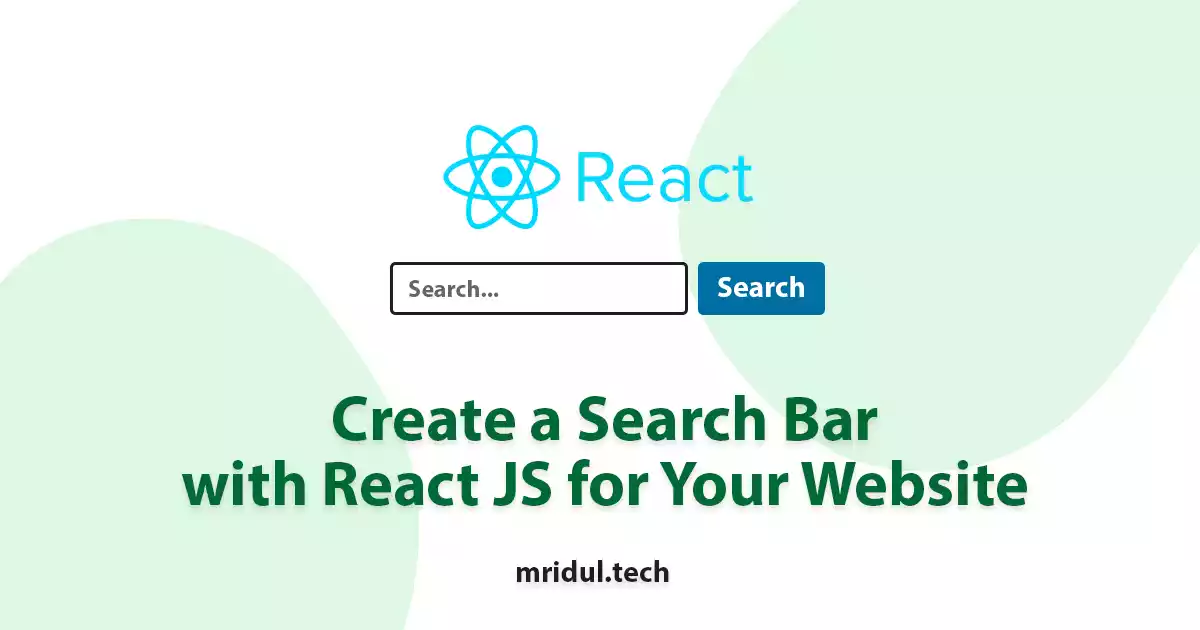
A search bar is an essential component of any website, allowing visitors to find the information they need quickly. If you are building a website using React, you can easily create a search bar to improve the user experience. In this article, we will guide you through the process of creating a React search bar step-by-step. By the end of this guide, you will have a fully functional search bar that can be integrated into your website.
Prerequisites for the Search Bar
Before we start, you should have a basic understanding of React and JavaScript. You will need to have Node.js and npm installed on your computer. You can download them from the official Node.js website if you don’t have them installed.
If you are looking for the code, You can find the code for this project on GitHub.
Setting up the React Project
To create a React search bar, you will need to create a new React project. You can do this by running the following commands in your terminal:
npx create-react-app search-app
cd search-app
npm start
This will create a new React project called search-app
. Once the project is created, you can open it in your browser by navigating to http://localhost:3000
.
Also Read: Next JS vs React: Which One Should You Choose?
Creating the Search Bar Component
The next step is to create the search bar component. In your project directory, under src
folder create a new file called SearchBar.js
where we will add the search bar component.
// ./src/SearchBar.js
import React from 'react';
const SearchBar = () => {
return (
<form>
<input type="text" placeholder="Search..." />
<button type="submit">Search</button>
</form>
);
}
export default SearchBar;
In the above code, we have defined a functional component called SearchBar
. The component returns a form with an input field and a submit button. We have also added a placeholder text to the input field.
Also Read – How to Fix It React Hook useEffect Has a Missing Dependency
Adding Styles in the Search Bar
To make the search bar look more appealing, we can add some styles to it. Create a new file called SearchBar.css
in your project directory and add the following code:
// ./src/SearchBar.css
form {
display: flex;
align-items: center;
justify-content: center;
margin-bottom: 1rem;
width: 100%;
}
input[type="text"] {
padding: 0.5rem;
border-radius: 0.25rem;
border: none;
box-shadow: 0 1px 3px rgba(0, 0, 0, 0.2);
border: 1px solid rgba(0, 0, 0, 0.2);
width: 100%;
max-width: 250px;
margin-right: 0.5rem;
}
button[type="submit"] {
padding: 0.5rem;
background-color: #007bff;
color: #fff;
border: none;
border-radius: 0.25rem;
cursor: pointer;
}
In the above code, we have defined some basic styles for the search bar. We have used flexbox to align the input field and the submit button. We have also added some padding and border-radius to the input field to make it look more appealing. The submit button has a blue background color and white text.
Also Read: Top 5 Free APIs for Your Next Project
Adding Functionality to the Search Bar
Now that we have created and styled the search bar, we need to add some functionality to it. We will use React hooks to add functionality to the search bar component.
import React, { useState } from 'react';
const SearchBar = () => {
const [searchQuery, setSearchQuery] = useState('');
const handleSearchInputChange = (event) => {
setSearchQuery(event.target.value);
}
const handleSearchSubmit = (event) => {
event.preventDefault();
console.log(`Searching for: ${searchQuery}`);
// Add your search functionality here
}
return (
<form onSubmit={handleSearchSubmit}>
<input
type="text"
placeholder="Search..."
value={searchQuery}
onChange={handleSearchInputChange}
/>
<button type="submit">Search</button>
</form>
);
}
export default SearchBar;
In the above code, we have added two new functions to the component: handleSearchInputChange
and handleSearchSubmit
. The handleSearchInputChange
function updates the search query state whenever the user types something in the input field. The handleSearchSubmit
function is called when the user clicks the search button or presses Enter.
When the form is submitted, the handleSearchSubmit
function logs the search query to the console. You can add your own search functionality here, such as making an API call to fetch search results and display them on the page.
Also Read: Top 5 Websites for Free and Beautiful Stock Images
Search Functionality Logic
To check our search functionality we are going to use some Pokemon Data. Initially, we will render all the data initially, but when we click on the search button the filtered data will be rendered. For that, we will import the data in App.js
file.
// ./src/App.js
import React, { useState } from "react";
import SearchBar from "./SearchBar";
import { pokedata as data } from "./data";
import "./App.css";
const App = () => {
const [filteredData, setFilteredData] = useState(data);
return (
<div className="container">
<SearchBar
data={data}
setFilteredData={setFilteredData}
/>
<div className="pokedex">
{filteredData.map((pokemon) => (
<div className="pokemon" key={pokemon.id}>
<div>{pokemon.name}</div>
<div>{pokemon.type}</div>
</div>
))}
</div>
</div>
);
};
export default App;
Here we have that SearchBar component that takes in two props: data
and setFilteredData
. The data prop is an array of objects that we want to filter through. The setFilteredData
prop is a function that we can call to update the filteredData
state in our App component. We can then use the filteredData
state to render the filtered data in our App component.
We have also mapped over the filteredData
state in our App component to render the filtered data. Also, We are using the id property of each object in the array as the key for each rendered element.
The styles for the mapped components are here –
// ./src/App.css
.container {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
padding: 2rem;
}
.pokedex {
display: flex;
align-items: center;
justify-content: center;
flex-wrap: wrap;
}
.pokemon {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
margin: 1rem;
border: 1px solid black;
border-radius: 1rem;
width: 8rem;
height: 8rem;
}
Here is the function for the handleSearchSubmit
with the search logic. The value of the input field is set to the searchQuery
state variable. This will allow the user to see what they are typing in the input field.
const handleSearchSubmit = (event) => {
event.preventDefault();
console.log(`Searching for: ${searchQuery}`);
const filteredData = data.filter((pokemon) => {
return pokemon.name.toLowerCase().includes(searchQuery.toLowerCase());
});
setFilteredData(filteredData);
};
The code for this project is on GitHub
Conclusion
Creating a search bar in React is a simple and straightforward process. You can create a fully functional search bar with the following steps and add it to your website’s navbar. Remember to use React hooks to add functionality to your search bar component, and to style your search bar to make it look more appealing. Happy coding!
You may also like
React Hooks Cheatsheet
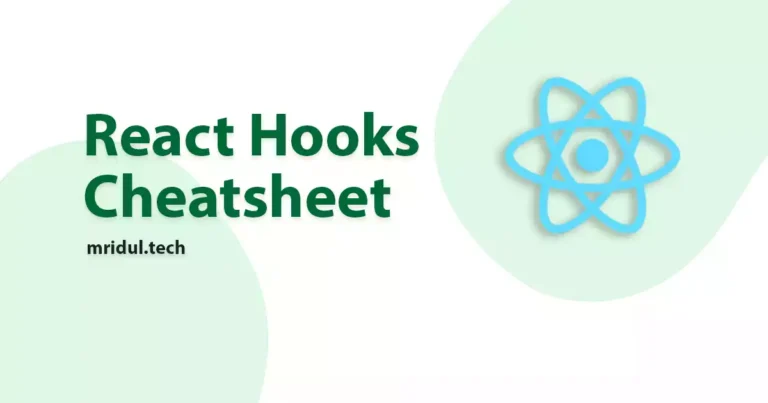
Jan 21, 2024
·4 Min Read
React, a popular JavaScript library for building user interfaces, introduced Hooks to make functional components more powerful and expressive. Let’s dive into the React Hooks Cheatsheet to understand how they enhance the development experience. React Hooks Cheatsheet In the ever-evolving landscape of web development, React Hooks have become indispensable for building dynamic and efficient user […]
Read More
React Emoji Picker: Add Emoji Picker in a React App
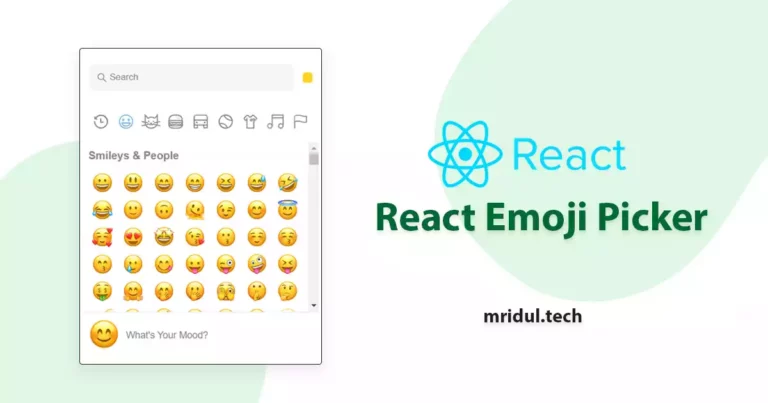
May 18, 2023
·3 Min Read
Emojis have become a staple in modern communication, with people using them to convey emotions and ideas in various contexts. As such, it’s important for developers to provide users with an easy and intuitive way to input emojis into their applications. This is where the React Emoji Picker comes in handy. This tool simplifies the […]
Read More
Creating a Stunning Image Gallery using React Image Gallery
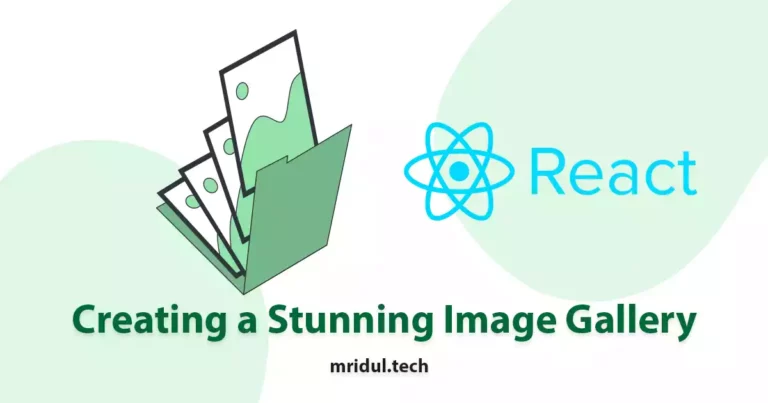
Apr 19, 2023
·5 Min Read
When it comes to building a website, a visually appealing image gallery can make a huge difference in the overall user experience. React Image Gallery is a popular and flexible Reactjs component library that can help you create a stunning gallery for your website. In this article, we will explore the features of React Image […]
Read More
Top 5 Platforms to Host React Apps for Free
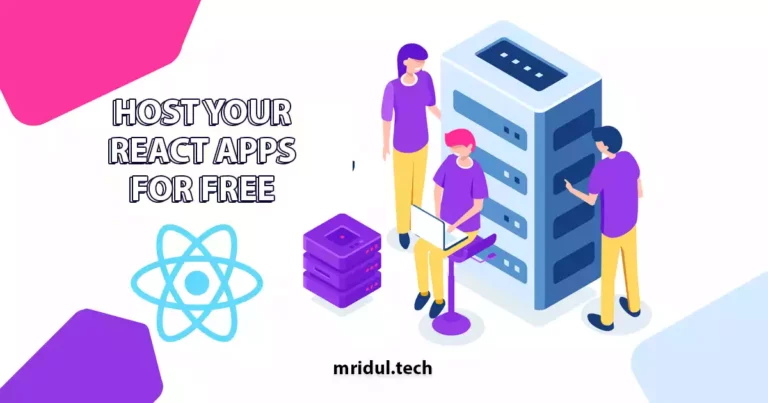
Apr 11, 2023
·7 Min Read
Learn about the best platforms to host your React apps for free. This guide will help you choose the right platform that suits your needs.
Read More
How to Upload Images to Cloudinary With React JS
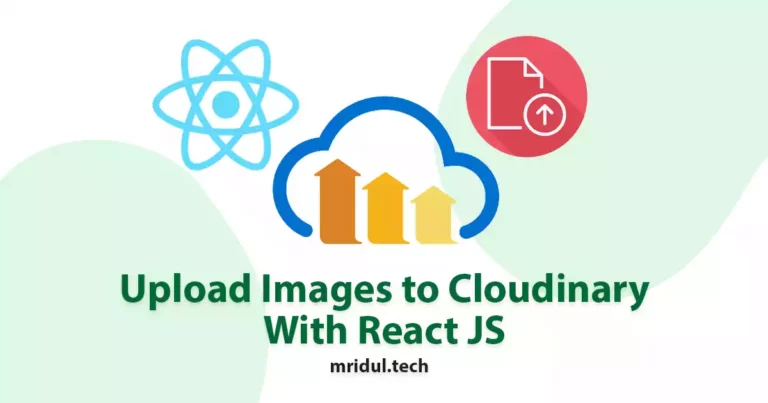
Apr 05, 2023
·6 Min Read
Are you struggling to upload images in your React JS application? Are you tired of the tedious process of configuring file uploads and handling image storage? If so, you are not alone. Many developers find image management in web applications to be a challenge. Fortunately, Cloudinary offers an easy solution. In this article, we will […]
Read More
React Hook useEffect Has a Missing Dependency: How to Fix It
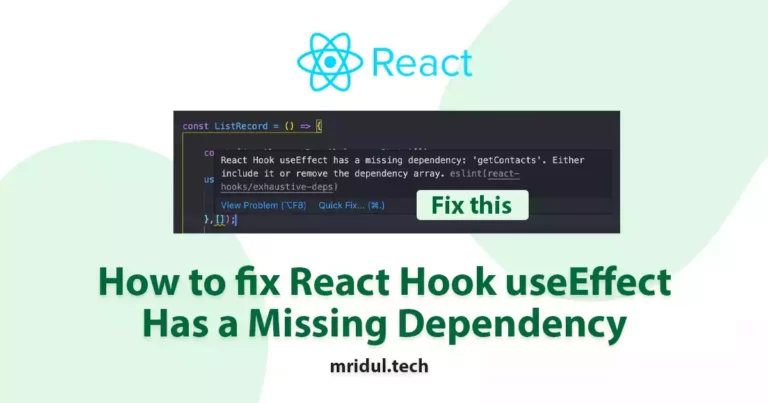
Mar 10, 2023
·6 Min Read
React is one of the most popular JavaScript libraries used for building user interfaces. One of the main advantages of React is the use of hooks, which allow you to use state and other React features in functional components. One of the most commonly used hooks is useEffect, which is used for handling side effects […]
Read More