React Hooks Cheatsheet
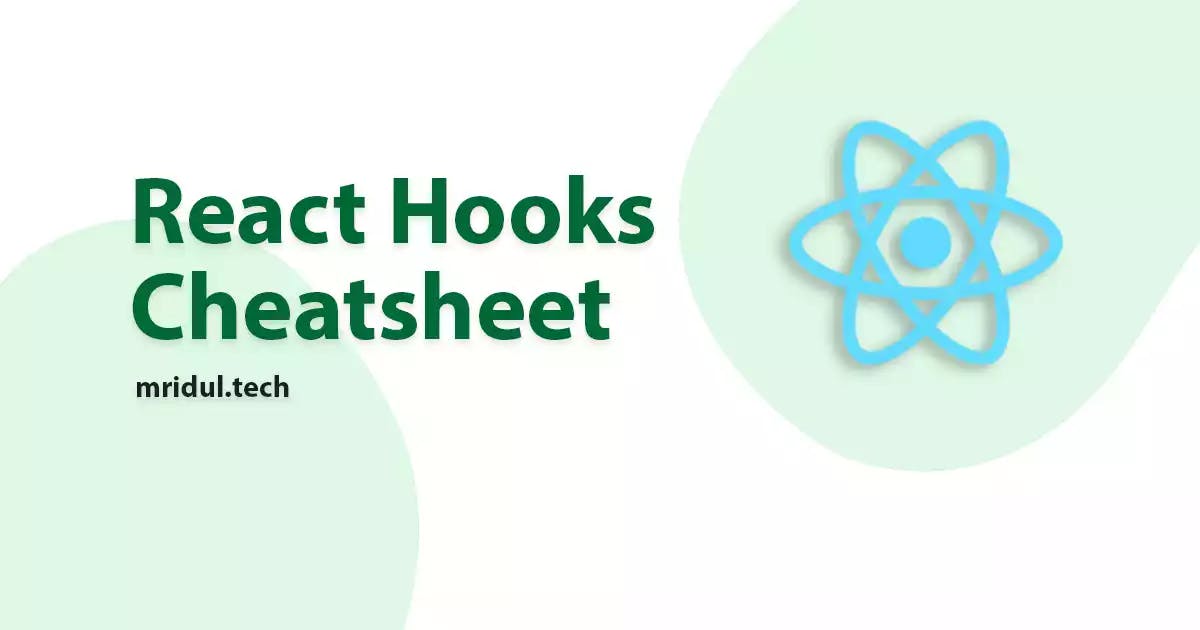
React, a popular JavaScript library for building user interfaces, introduced Hooks to make functional components more powerful and expressive. Let’s dive into the React Hooks Cheatsheet to understand how they enhance the development experience.
React Hooks Cheatsheet
In the ever-evolving landscape of web development, React Hooks have become indispensable for building dynamic and efficient user interfaces. Hooks allow developers to manage state, handle side effects, and reuse logic in functional components. This article serves as a comprehensive guide and cheatsheet for mastering React Hooks.
Also Read: React Emoji Picker: Add Emoji Picker in a React App
State Hooks
State Hooks are used to manage state in functional components. They allow us to add local state to our function components.
useState Hook
useState
declares a state variable that can be immediately updated and assigned to any type of data.
const [search, setSearch] = useState("");
useReducer Hook
useReducer
declares a state variable inside a reducer function with update logic
const [state, dispatch] = useReducer(counterReducer, { count: 0 });
Also Read: Creating a Stunning Image Gallery using React Image Gallery
Context Hooks
Context Hooks are used to share state between components without having to pass props down manually at every level.
As an example, the top-level component of your application can transmit the current UI style to all components, regardless of depth, below it.
React context allows you to prevent “prop drilling,” which is the practice of transferring data from one component to its child and then from that child component to another child component.
Every component that subscribes to the context receives all of the data with context.
useContext Hook
useContext
reads and subscribes to a context
const theme = useContext(ThemeContext);
Ref Hooks
Ref Hooks are used to access DOM nodes or React elements created in the render method. They allow us to access DOM nodes or React elements created in the render method.
useRef Hook
useRef
declares a ref and you can hold any value in it
const inputRef = useRef();
useImperativeHandle Hook
useImperativeHandle
lets you customize the ref exposed by the component. This hook is rarely used
useImperativeHandle(ref, () => {
})
Effect Hooks
Effect Hooks are used to perform side effects in function components. They allow us to perform side effects in function components.
useEffect Hook
useEffect
essentially allows you to implement side effects in functional components. This covers communication with external systems such as data retrieval, subscriptions, manual DOM manipulation, and so forth.
useEffect(() => {
const unsubscribe = socket.connect(userId);
return () => {
unsubscribe();
};
}, [userId]);
useLayoutEffect Hook
With useLayoutEffect
we can read DOM layout and do DOM mutations before the browser paints.
useLayoutEffect(() => {
// Read DOM layout
});
useInsertionEffect Hook
with useInsertionEffect
we can do DOM mutations after the browser paints.
useInsertionEffect = () => {
// Do DOM mutations here
}
Also Read: How to Upload Images to Cloudinary With React JS
Subscribing Hooks
Subscribing Hooks are used to subscribe to external data sources and update our state when they change.
useSyncExternalStore Hook
With useSyncExternalStore
we can subscribe to external data sources and update our state when they change.
useSyncExternalStore = () => {
// Subscribe to external data source
// Update state when data changes
}
Memoization Hooks
Memoization Hooks are used to optimize expensive calculations. They allow us to cache the result of a function and only recompute it if its dependencies change.
useCallback Hook
With useCallback
Hook we can cache the result of a function and only recompute it if its dependencies change.
const memoizedCallback = useCallback(() => {
doSomething(a, b);
}, [a, b]);
useMemo Hook
With useMemo
Hook we can cache the result of a function and only recompute it if its dependencies change.
const memoizedValue = useMemo(() => {
return computeExpensiveValue(a, b), [a, b];
});
Also Read: How to Create a React Search Bar for Your Website
Accessibility Hooks
Accessibility Hooks are used to make our apps more accessible to users with disabilities.
useId Hook
With useId
Hook we can generate a unique ID that is guaranteed to be the same between renders.
const id = useId();
Debug Hooks
Debug Hooks are used to debug our custom Hooks. These hooks are not meant to be used in production code.
useDebugValue Hook
With useDebugValue
Hook we can label our custom Hooks to make them easier to debug.
useDebugValue('my custom hook');
Events Hooks
Events Hooks are used to handle events in function components. They allow us to handle events in function components.
useEvent Hook
With useEvent
Hook we can extract non-reactive effect logic into an event function.
useEvent(callback)
Transition Hooks
Transition Hooks are used to mark updates as transitions. They allow us to mark updates in the provided callback as transitions.
useTransition Hook
With useTransition
Hook we can mark updates in the provided callback as transitions.
startTransition (() => {
setCount ( count + 1);
})
useDeferredValue Hook
With useDeferredValue
Hook we can defer to more urgent updates.
const deferredValue = useDeferredValue(value);
Conclusion: React Hooks Cheatsheet
In conclusion, the React Hooks Cheatsheet serves as a comprehensive resource for developers looking to master the art of functional component development. By embracing React Hooks, developers can streamline their workflow, create more maintainable code, and stay ahead in the dynamic world of web development.
You may also like
React Emoji Picker: Add Emoji Picker in a React App

May 18, 2023
·3 Min Read
Emojis have become a staple in modern communication, with people using them to convey emotions and ideas in various contexts. As such, it’s important for developers to provide users with an easy and intuitive way to input emojis into their applications. This is where the React Emoji Picker comes in handy. This tool simplifies the […]
Read More
Creating a Stunning Image Gallery using React Image Gallery

Apr 19, 2023
·5 Min Read
When it comes to building a website, a visually appealing image gallery can make a huge difference in the overall user experience. React Image Gallery is a popular and flexible Reactjs component library that can help you create a stunning gallery for your website. In this article, we will explore the features of React Image […]
Read More
Top 5 Platforms to Host React Apps for Free

Apr 11, 2023
·7 Min Read
Learn about the best platforms to host your React apps for free. This guide will help you choose the right platform that suits your needs.
Read More
How to Upload Images to Cloudinary With React JS

Apr 05, 2023
·6 Min Read
Are you struggling to upload images in your React JS application? Are you tired of the tedious process of configuring file uploads and handling image storage? If so, you are not alone. Many developers find image management in web applications to be a challenge. Fortunately, Cloudinary offers an easy solution. In this article, we will […]
Read More
How to Create a React Search Bar for Your Website
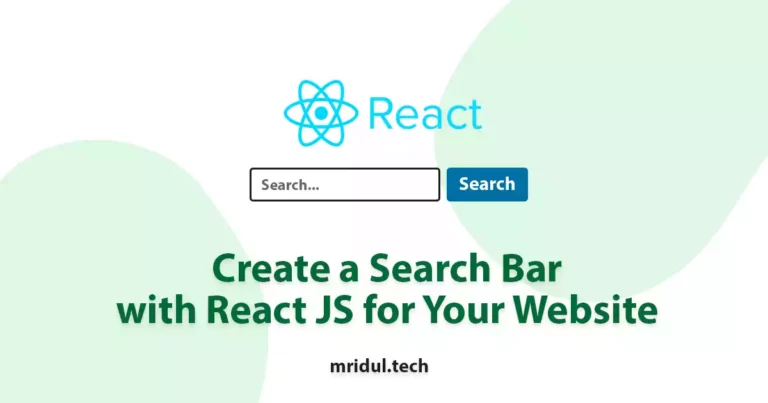
Mar 18, 2023
·6 Min Read
A search bar is an essential component of any website, allowing visitors to find the information they need quickly. If you are building a website using React, you can easily create a search bar to improve the user experience. In this article, we will guide you through the process of creating a React search bar […]
Read More
React Hook useEffect Has a Missing Dependency: How to Fix It

Mar 10, 2023
·6 Min Read
React is one of the most popular JavaScript libraries used for building user interfaces. One of the main advantages of React is the use of hooks, which allow you to use state and other React features in functional components. One of the most commonly used hooks is useEffect, which is used for handling side effects […]
Read More