How to Create Heartbeat Animation in CSS

With only HTML and CSS you can create a lot of create animation and a lot of shapes in the web. And in this article you will learn how to draw a heart shape and add Heartbeat Animation in CSS to the shape
Get the full source code of the heartbeat animation on GitHub
Draw a Heart with CSS
First we will learn how we can draw a heart with pure CSS. The heart shape contains two round corner and slanting lines at the bottom. For this we will need only one div in the HTML.
// index.html
<div class="heart"></div>
1. We will give that div a hight and width of 100px
. Then it will be squre of 100px
in each side.
2. Now we will rotate the squre 45deg
so that we can get the slanting lines at the bottom. This square will appear to be inverted, like a diamond.
// styles.css
.heart {
position: relative;
// Square with size of 100px
width: 100px;
height: 100px;
background-color: #ff3838;
// Rotate 45 deg to look like a diamond
transform: rotate(45deg);
}
3. Now we will create a similar-sized pseudo element with a 50% border radius. These pseudo elements are behind the Heart container and won’t be visible. In order to make the elements visible we need to give top and left position to both the elements. The :after
will be used for right side of the heart and :before
for the left side. Both pseudo elements will be circles. The
// styles.css
.heart:before,
.heart:after {
content: "";
position: absolute;
top: 0;
left: 0;
// Pseudo elements of same size
width: 100%;
height: 100%;
// Same background color as the div
background-color: #ff3838;
// Full rounded borders
border-radius: 50%;
}
// The before element will have the translateY
.heart:before {
transform: translateY(-50%);
}
// The after element will have the translateX
.heart:after {
transform: translateX(-50%);
}
Also Read: How to add input tags in HTML
Heartbeat Animation in CSS
4. First we will create a keyframe animation for the CSS. Using the transform property, we will scale the heart to larger and smaller sizes at various intervals. This will be our heartbeat keyframe.
@keyframes heartbeat {
0% { transform: rotate(45deg) scale(1); }
25% { transform: rotate(45deg) scale(1); }
30% { transform: rotate(45deg) scale(1.4); }
50% { transform: rotate(45deg) scale(1.2); }
70% { transform: rotate(45deg) scale(1.4); }
100% { transform: rotate(45deg) scale(1); }
}
5. Now we will apply the heartbeat keyframe to the container to create an infinite animation.
.heart {
// animation: <animation-name> <animation-duration> <animation-iteration-count>
animation: heartbeat 1.4s linear infinite;
}
Also Learn: How to use CSS Animations with Styled Components
Keyframe Animation Explaintion
<animation-name>
: Theheartbeat
keyframe which we defined in the previous step is theanimation-name
<animation-duration>
: This is how long do we need to run each animation. If we define 2s, then our animation will run for 2s.<animation-iteration-count>
: This defines how many time our animation will run. If we define this as infinite, then it will run the animation infinitely after every X seconds which is defined in theanimation-duration
The full source code of the heartbeat animation on GitHub. The Final output will look like this.
// Full CSS Code
.heart {
position: relative;
width: 100px;
height: 100px;
background-color: #ff3838;
transform: rotate(45deg);
animation: heartbeat 1.4s linear infinite;
}
.heart:before,
.heart:after {
content: "";
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-color: #ff3838;
border-radius: 50%;
}
.heart:before {
transform: translateY(-50%);
}
.heart:after {
transform: translateX(-50%);
}
@keyframes heartbeat {
0% {
transform: rotate(45deg) scale(1);
}
25% {
transform: rotate(45deg) scale(1);
}
30% {
transform: rotate(45deg) scale(1.4);
}
50% {
transform: rotate(45deg) scale(1.2);
}
70% {
transform: rotate(45deg) scale(1.4);
}
100% {
transform: rotate(45deg) scale(1);
}
}
You may also like
Top 5 CSS Libraries for Web Developers in 2023
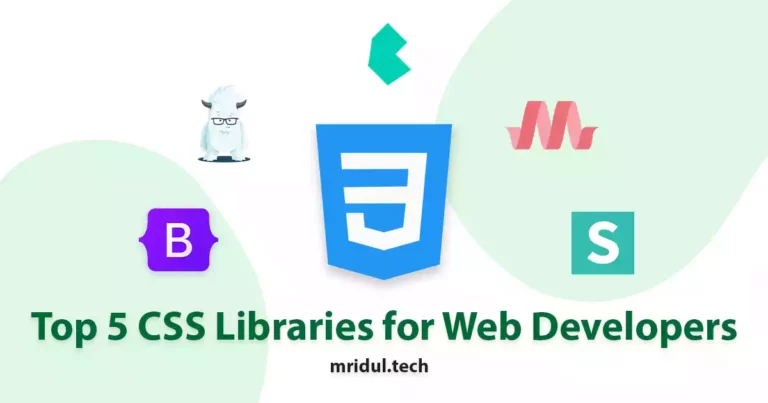
Mar 30, 2023
·6 Min Read
CSS or Cascading Style Sheets is an essential part of modern web development. It enables developers to create visually appealing and responsive websites that engage users. However, developing CSS from scratch can be time-consuming and challenging. Fortunately, there are numerous CSS libraries available that make CSS development more manageable, efficient, and effective. In this article, […]
Read More
How to use CSS Animations with Styled Components
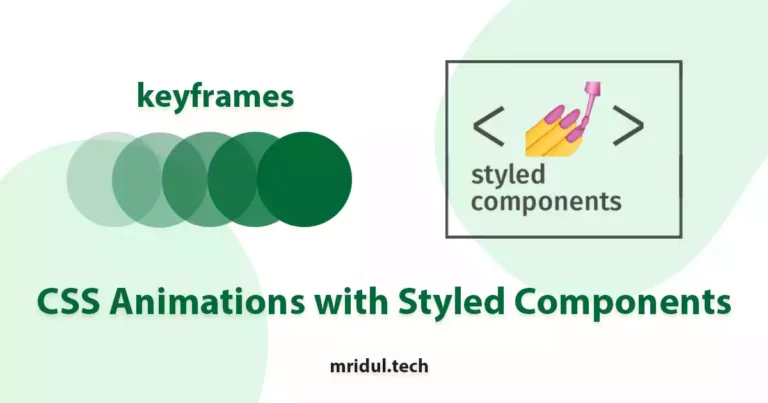
Jan 30, 2023
·3 Min Read
The styled component is one of the most popular libraries for giving styles to React JS and Next JS projects. It has over 4.9 Million downloads in NPM. With styled-components, we can write the CSS in the component file, providing so much maintainable and clean code. But when you try to add CSS keyframes the […]
Read More