How to add Grid Layout in React Native
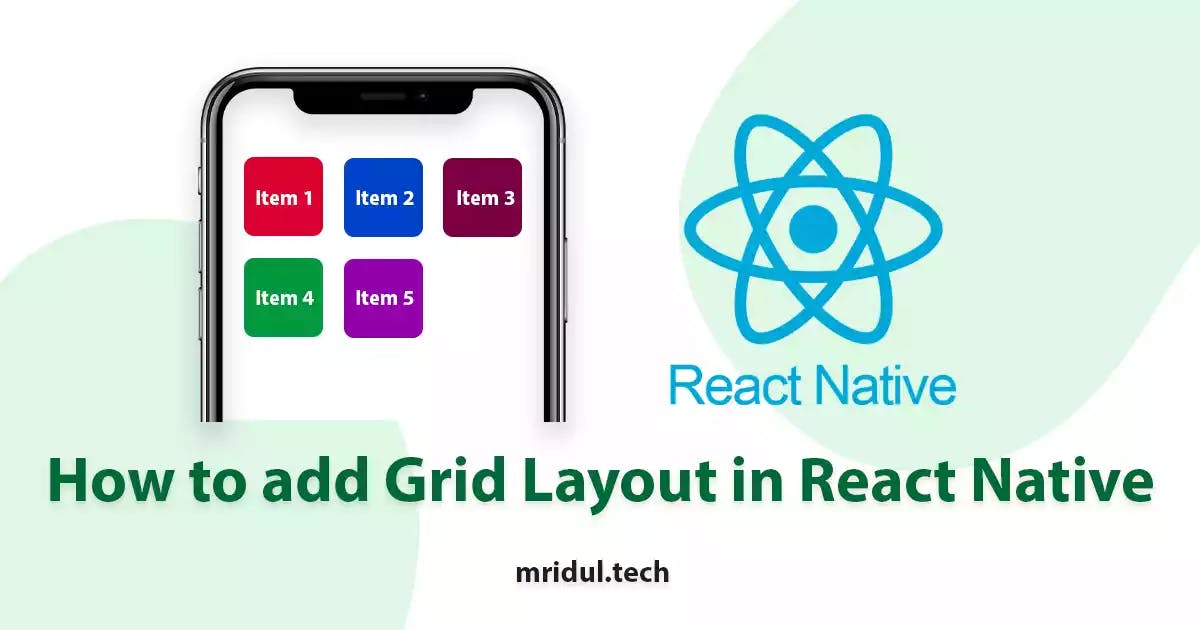
It is easy to create a grid layout css
as it supports display: grid
and with this, we can create a grid layout. We can even set an even spacing between items. Also, we can arrange a specific number of items in a column. But all of this is unsupported in React Native styles. So it is hard to create a layout like this in React Native. In this article, we are going to make a Grid Layout in React Native, which will be the same visually as the CSS grid.
Initialize Grid Layout
For this, we are not going to install any package. We are going to create it from scratch with React Native Stylesheet. So let’s create this step by step. The full code will be at the end of the article.
- First, we need to analyze the width of the user screen. For this, we are going to import the
Dimensions
from react native package.
import { Dimensions } from 'react-native';
- Then we will get the width of the screen
// Get the width of screen
const { width } = Dimensions.get('window');
const windowWidth = width;
- For this article, we will take 3 items per row and the gap will be 12 units. We will save these two values in two variables so that we can use it later as well
const gap = 12;
const itemPerRow = 3;
- The total gap will be 2 for 3 items. so for our example, the total gap is 12*2 = 24 units.
const totalGapSize = (itemPerRow - 1) * gap;
- So each child will get a space of total available width after removing the total gap width / 3
const childWidth = (windowWidth - totalGapSize) / itemPerRow;
Grid Layout in React Native Component
For the component, we are going to add some dummy data in a react-native scroll view. So that this layout can update dynamically with as many items as we can provide. Let’s create the map with dummy data.
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView } from 'react-native';
const GridView = () => {
const items = [
{
id: 1,
title: 'Item 1',
backgroundColor: 'red',
},
{
id: 2,
title: 'Item 2',
backgroundColor: 'green',
},
{
id: 3,
title: 'Item 3',
backgroundColor: 'blue',
},
{
id: 4,
title: 'Item 4',
backgroundColor: 'yellow',
},
{
id: 5,
title: 'Item 5',
backgroundColor: 'orange',
},
];
return (
<SafeAreaView>
<ScrollView>
{items.map((item) => (
<Text
key={item.id}
style={{
backgroundColor: item.backgroundColor,
}}
>
{item.title}
</Text>
))}
</ScrollView>
</SafeAreaView>
);
};
export default GridView;
const styles = StyleSheet.create({});
Also Read: How to Change React Native App Icon for iOS and Android
Now we are going to add some styles to the Scrollview and to the items. First, add the styles for the container. The styles will be the following
itemsWrap: {
display: 'flex',
flexDirection: 'row',
flexWrap: 'wrap',
marginVertical: -(gap / 2),
marginHorizontal: -(gap / 2),
},
Look how we are subtracting half of the gap size from the margin. This is the important part. For the child items styles, these will be styles
singleItem: {
marginHorizontal: gap / 2,
minWidth: childWidth,
maxWidth: childWidth,
},
For a single item, we are adding the half of gap marginHorizontal
to normalize the spacing between items. And here is the full code –
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView } from 'react-native';
// Gap stuff
const { width } = Dimensions.get('window');
const gap = 12;
const itemPerRow = 3;
const totalGapSize = (itemPerRow - 1) * gap;
const windowWidth = width;
const childWidth = (windowWidth - totalGapSize) / itemPerRow;
const GridView = () => {
const items = [
{
id: 1,
title: 'Item 1',
backgroundColor: 'red',
},
{
id: 2,
title: 'Item 2',
backgroundColor: 'green',
},
{
id: 3,
title: 'Item 3',
backgroundColor: 'blue',
},
{
id: 4,
title: 'Item 4',
backgroundColor: 'yellow',
},
{
id: 5,
title: 'Item 5',
backgroundColor: 'orange',
},
];
return (
<SafeAreaView>
<ScrollView style={styles.itemsWrap}>
{items.map((item) => (
<Text
key={item.id}
style={{
backgroundColor: item.backgroundColor,
...styles.singleItem,
}}
>
{item.title}
</Text>
))}
</ScrollView>
</SafeAreaView>
);
};
export default GridView;
const styles = StyleSheet.create({
itemsWrap: {
display: 'flex',
flexDirection: 'row',
flexWrap: 'wrap',
marginVertical: -(gap / 2),
marginHorizontal: -(gap / 2),
},
singleItem: {
marginHorizontal: gap / 2,
minWidth: childWidth,
maxWidth: childWidth,
},
});
You may also like
How to reduce React Native APK Size?

Feb 18, 2024
·2 Min Read
In the ever-evolving landscape of mobile app development, optimizing React Native APK size is crucial for delivering a seamless user experience. Developers often grapple with bloated APKs, hindering app downloads and user retention. This comprehensive guide unveils practical steps to efficiently reduce React Native APK size, ensuring your application remains agile and user-friendly. Also Read: […]
Read More
How to use SVG in React Native?

Feb 02, 2024
·3 Min Read
In the ever-evolving world of mobile app development, React Native has emerged as a popular framework for building cross-platform applications. One of the intriguing aspects of React Native is its support for Scalable Vector Graphics (SVG). In this article, we’ll delve into the intricacies of rendering SVG in React Native and explore best practices to […]
Read More
How to change Status Bar Background Color in React Native

Jan 12, 2024
·3 Min Read
In the dynamic world of mobile app development, creating a seamless and engaging user experience is paramount. One often-overlooked aspect that can significantly impact the overall feel of a React Native application is the status bar. This article will guide you on how to change the status bar background color in React Native, allowing you […]
Read More
How to Change React Native App Icon for iOS and Android

Jan 22, 2023
·4 Min Read
The Application Icon provides the App’s unique identifier. The primary thing that users usually remember is the application icon. Most of the time, a user can recall an application’s symbol rather than its name. The objective of your application should be defined by the app icon, which might be your brand’s logo or anything else. […]
Read More