Anonymous Functions in JavaScript
Mridul Panda
Mar 21, 2023
·4 Min Read
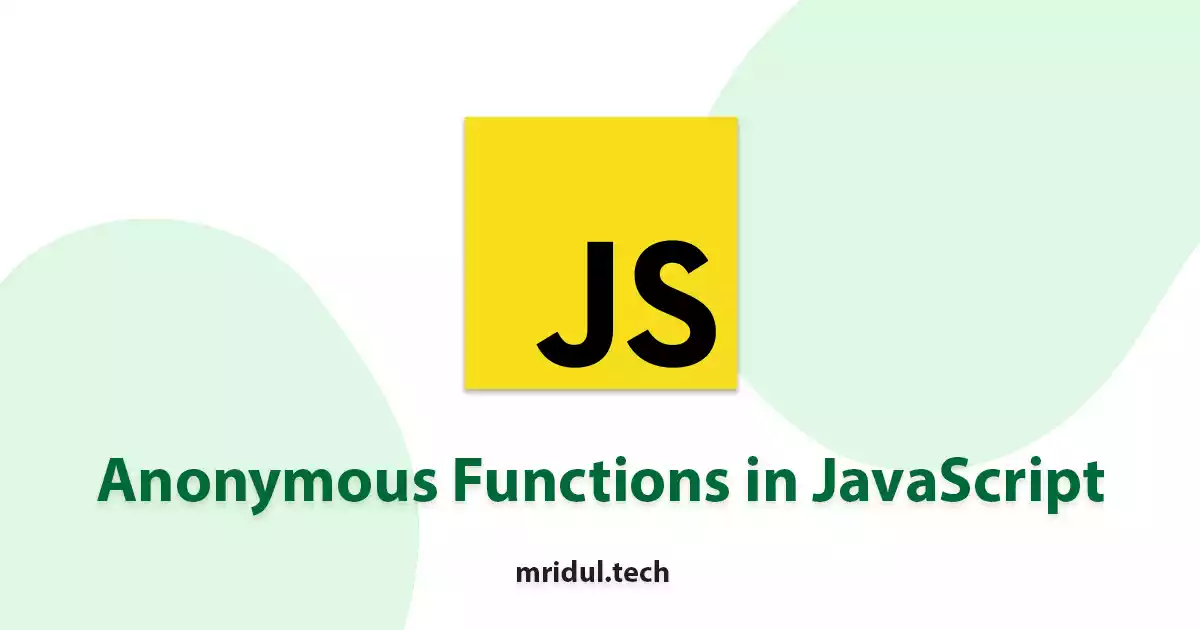
JavaScript is a dynamic programming language that has grown significantly in popularity over the years. It offers several features that make it one of the most flexible programming languages around. One of these features is anonymous functions. In this article, we will take a closer look at anonymous functions in JavaScript, including what they are, how they work, and why they are so useful.
What are Anonymous Functions in JavaScript?
An anonymous function, also known as a lambda function or function expression, is a function that does not have a name. In JavaScript, anonymous functions are created using the function keyword followed by a set of parentheses, which contain the function parameters, and then a block of code that makes up the function body.
Anonymous functions are not defined in the global scope, and they do not have a function name that can be used to call them. Instead, they are often assigned to variables, passed as arguments to other functions, or used as part of different expressions.
Advantages of Using Anonymous Functions
There are several advantages to using anonymous functions in JavaScript:
- Modularity: Anonymous functions can be used to create modular code that is easier to read and maintain.
- Encapsulation: Anonymous functions can be used to create private functions that are only accessible within the scope of the outer function.
- Asynchronous programming: Anonymous functions can be used to create callback functions that are executed when an asynchronous operation is completed.
Common Use Cases for Anonymous Functions
Anonymous functions are used extensively in JavaScript and are an important part of the language. Some common use cases for anonymous functions include:
- Event handlers: Anonymous functions can be used to handle events such as mouse clicks, button presses, and key presses.
- Callback functions: Anonymous functions can be used as callback functions for asynchronous operations such as AJAX requests or setTimeout functions.
- Iterators: Anonymous functions can be used as iterators for arrays, maps, and other data structures.
- Immediately invoked function expressions (IIFE): Anonymous functions can be used to create self-invoking functions that run immediately when they are defined.
Defining anonymous functions
Anonymous functions can be defined in two ways. The first way is to use the function keyword followed by a set of parentheses and curly braces. The second way is to use an arrow function.
// Using the function keyword
let anonymousFunction = function() {
console.log("This is an anonymous function.");
};
// Using arrow functions (ES6)
let anonymousFunction = () => {
console.log("This is an anonymous function.");
};
Also Read: How to add input tags in HTML
Using anonymous functions as callbacks
One of the most common ways to use anonymous functions is as callbacks. A callback function is a function that is passed as an argument to another function and is executed after some operation has been completed. Here is an example of using an anonymous function as a callback.
let numbers = [1, 2, 3, 4, 5];
numbers.forEach(function(number) {
console.log(number * 2);
});
In this example, we are using an anonymous function as the callback for the forEach method. The anonymous function takes a single argument, which represents each item in the array.
Also Read: Top 5 Free APIs for Your Next Project
Passing arguments to anonymous functions
Anonymous functions can also take arguments just like regular functions. These arguments can be passed in when the function is defined or when it is called.
let anonymousFunction = function(arg1, arg2) {
console.log(arg1 + arg2);
};
anonymousFunction(2, 3); // Output: 5
Also Read: How to handle Cookie Consent in Next JS
Using anonymous functions with IIFE
IIFE stands for Immediately Invoked Function Expression. It is a function that is defined and executed at the same time. Anonymous functions are often used in IIFE because they do not need to be named. Here is an example of an IIFE using an anonymous function.
(function() {
console.log("This is an immediately invoked anonymous function.");
})();
Arrow functions in ES6
Arrow functions were introduced in ES6 as a more concise way to define anonymous functions. They use a shorthand syntax that makes them easier to read and write. Here is an example of an arrow function.
let anonymousFunction = (arg1, arg2) => {
console.log(arg1 + arg2);
};
anonymousFunction(2, 3); // Output: 5
In this example, the arrow function takes two arguments and returns their sum. The =>
symbol separates the function parameters from the function body.
Also Read: How to add Google AdSense in Next JS
Conclusion
In conclusion, anonymous functions are a powerful tool in JavaScript that allows for more modular, encapsulated, and asynchronous code. They are commonly used for event handlers, callbacks, iterators, and immediately invoked function expressions. Named function expressions and arrow functions are alternative ways to define functions in JavaScript. However, anonymous functions also have limitations, including difficulties with debugging, readability, and testing. By understanding the benefits and limitations of anonymous functions, developers can make informed decisions about when to use them in their code.